https://www.byteslounge.com/tutorials/jaas-authentication-in-tomcat-example
Introduction
Tomcat provides a default JAAS Realm implementation so developers may implement JAAS Login Modules and easily integrate them with the container. In this tutorial we will implement all the required components to put JAAS up and running in Tomcat web container.
This tutorial considers the following software and environment:
- Ubuntu 12.04
- JDK 1.7.0.09
- Tomcat 7.0.35
The Principals
One of the core concepts of JAAS is the existence of users and roles (roles are similar to groups in UNIX systems). Authorization may be issued to specific users or to roles. In JAAS this is concept is translated to Principals: Principals may represent users or roles independently. Let's define User and Role Principals to be used in this example:
package com.byteslounge.jaas;import java.security.Principal;publicclassUserPrincipalimplementsPrincipal{privateString name;publicUserPrincipal(String name){super();this.name = name;}publicvoid setName(String name){this.name = name;}@OverridepublicString getName(){return name;}}
package com.byteslounge.jaas;import java.security.Principal;publicclassRolePrincipalimplementsPrincipal{privateString name;publicRolePrincipal(String name){super();this.name = name;}publicvoid setName(String name){this.name = name;}@OverridepublicString getName(){return name;}}
Basically we are defining two simple Principals, each one of them requiring just a name so they may be promptly identified (a username or a role name). Remember that our principals must implement the java.security.Principal interface.
The Login Module
Now we need to define a Login Module that will actually implement the authentication process. The Login module must implement the javax.security.auth.spi.LoginModule interface:
package com.byteslounge.jaas;import java.io.IOException;import java.util.ArrayList;import java.util.List;import java.util.Map;import javax.security.auth.Subject;import javax.security.auth.callback.Callback;import javax.security.auth.callback.CallbackHandler;import javax.security.auth.callback.NameCallback;import javax.security.auth.callback.PasswordCallback;import javax.security.auth.callback.UnsupportedCallbackException;import javax.security.auth.login.LoginException;import javax.security.auth.spi.LoginModule;publicclassBytesLoungeLoginModuleimplementsLoginModule{privateCallbackHandler handler;privateSubject subject;privateUserPrincipal userPrincipal;privateRolePrincipal rolePrincipal;privateString login;privateList<String> userGroups;@Overridepublicvoid initialize(Subject subject,CallbackHandler callbackHandler,Map<String,?> sharedState,Map<String,?> options){ handler = callbackHandler;this.subject = subject;}@Overridepublicboolean login()throwsLoginException{Callback[] callbacks =newCallback[2]; callbacks[0]=newNameCallback("login"); callbacks[1]=newPasswordCallback("password",true);try{ handler.handle(callbacks);String name =((NameCallback) callbacks[0]).getName();String password =String.valueOf(((PasswordCallback) callbacks[1]).getPassword());// Here we validate the credentials against some// authentication/authorization provider.// It can be a Database, an external LDAP, // a Web Service, etc.// For this tutorial we are just checking if // user is "user123" and password is "pass123"if(name !=null&& name.equals("user123")&& password !=null&& password.equals("pass123")){// We store the username and roles// fetched from the credentials provider// to be used later in commit() method.// For this tutorial we hard coded the// "admin" role login = name; userGroups =newArrayList<String>(); userGroups.add("admin");returntrue;}// If credentials are NOT OK we throw a LoginExceptionthrownewLoginException("Authentication failed");}catch(IOException e){thrownewLoginException(e.getMessage());}catch(UnsupportedCallbackException e){thrownewLoginException(e.getMessage());}}@Overridepublicboolean commit()throwsLoginException{ userPrincipal =newUserPrincipal(login); subject.getPrincipals().add(userPrincipal);if(userGroups !=null&& userGroups.size()>0){for(String groupName : userGroups){ rolePrincipal =newRolePrincipal(groupName); subject.getPrincipals().add(rolePrincipal);}}returntrue;}@Overridepublicboolean abort()throwsLoginException{returnfalse;}@Overridepublicboolean logout()throwsLoginException{ subject.getPrincipals().remove(userPrincipal); subject.getPrincipals().remove(rolePrincipal);returntrue;}}
All the implemented methods are inherited from javax.security.auth.spi.LoginModule interface and will be called by Tomcat at specific moments during the authentication process.
The login method is responsible for checking if the credentials provided by the end user are valid. This check is made against any kind of authorization entity: It may be a database, a web service, a LDAP, etc. The developer may implement this credentials check in the way required by some specific use case.
Note: The login method must throw a LoginException in case of authentication failure.
In the presence of a successful authentication it should fetch the roles associated with the authenticating user. In this case we simulated and hard coded the admin role as a fetched role from the credentials provider for the current user.
The commit method is called after a successful login method execution and is responsible to store the user and roles obtained by the login method in the respective Subject and in the form of Principals. As you can see in the above module implementation, during the login method execution the credentials are obtained by the means of a callback. This callback is initialized in the initialize method together with Subject initialization.
The logout method is called when the user logs out of the system (and the application implements a logout mechanism). Finally the abort method is called when the login method fails to authenticate the user (throws a LoginException).
The web application
In this example we will secure a specific folder of a Java web application. The application will be very simple and its structure is the following:
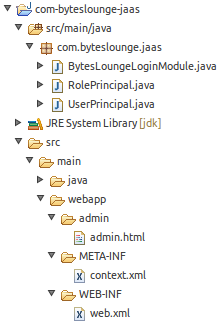
We will be securing the admin folder.
To accomplish this task we must define some configuration elements in web.xml file. These entries go directly under the web-app element:
<security-constraint><web-resource-collection><web-resource-name>Admin</web-resource-name><url-pattern>/admin/*</url-pattern></web-resource-collection><auth-constraint><role-name>admin</role-name></auth-constraint></security-constraint><security-role><role-name>admin</role-name></security-role><login-config><auth-method>BASIC</auth-method><realm-name>Admin</realm-name></login-config>
In security-constraint element we are defining that all resources under /admin folder are protected and only the admin role is granted to access the resources. All existing roles must be also defined in the security-role element. The login-config element defines how the credentials will be asked to the end user. In this example we will use the Basic authentication scheme (you may have different mechanisms like presenting a web form or page to the end user, but that will be covered in other tutorial).
Now we must define a new file named context.xml and place it under:
/META-INF/context.xml
<?xml version="1.0" encoding="UTF-8"?><Context><RealmclassName="org.apache.catalina.realm.JAASRealm"appName="BytesLoungeLogin"userClassNames="com.byteslounge.jaas.UserPrincipal"roleClassNames="com.byteslounge.jaas.RolePrincipal"/></Context>
Here we define the class that will implement the JAAS realm. We are using Tomcat default implementation: org.apache.catalina.realm.JAASRealm. We also define which classes will implement the user and roles Principals and we set them to be the ones we defined earlier in this tutorial (UserPrincipal and RolePrincipal). The attribute appName defines how the application will be globally identified by Tomcat in what matters to security configuration.
Finally we must define a JAAS configuration file. We will name it jaas.config and we will place it in Tomcat conf folder:
$CATALINA_BASE/conf/jaas.config
The file looks like the following:
BytesLoungeLogin{ com.byteslounge.jaas.BytesLoungeLoginModule required debug=true;};
This file defines the authentication configuration for BytesLoungeLogin application. Note that it's the same name we used in appName inside context.xml file just above.
Launching Tomcat and testing
Now lets launch Tomcat. We must set a JVM argument that tells Tomcat where the application configuration security file is located, the jaas.config file:
You may set this in the startup catalina.sh file.
When the server is up and running and we access the secure resource:
We will see the Basic authentication dialog asking for credentials:
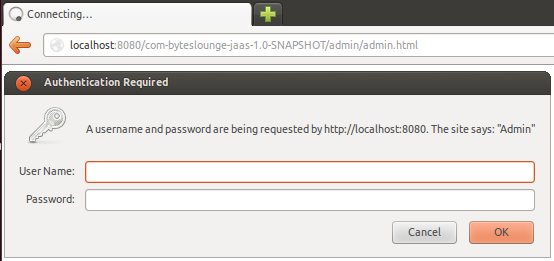
Now we insert the credentials we hard coded in our Login Module. Username: user123 and Password: pass123
We will be presented the secure resource. Access was granted.
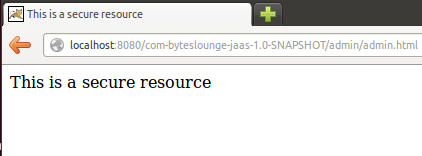
The tutorial full source code is available for download at the end of this page.
Logout process
For more information about the user logout process please refer to the following article:
Keep in mind that this tutorial covered BASIC authentication so your browser will store the user credentials until it's closed.
This means that even if you logout the user, as soon a new request is made against a protected resource the browser will send the credentials again and automatically authenticate the user.
If you need to definitely logout the user and force the credentials to be inserted again you should look into form based authentication: JAAS form based authentication in Tomcat example
相关推荐
**Java Authentication and Authorization Service (JAAS) 认证在Mac版Tomcat中的应用** Java Authentication and Authorization Service (JAAS) 是Java平台的核心组件,用于提供安全的用户认证和权限管理。在Mac版...
Java Authentication and Authorization Service (JAAS) 是Java平台中用于安全认证和授权的核心组件。它为开发者提供了一种标准的方式来管理用户的身份验证和访问控制,从而确保应用程序的安全性。在本精讲中,我们...
11. **安全性增强**:Servlet 2.5规范增强了安全特性,如支持JAAS(Java Authentication and Authorization Service)进行用户身份验证和权限控制。 12. **异步处理**:尽管Servlet 2.5并未直接提供异步处理能力,...
内容概要:报告由中国信息通信研究院发布,旨在评估制造业上市公司高质量发展,强调制造业高质量发展的重要性,并构建了涵盖创新力、竞争力、影响力、贡献力四大维度的评价体系。通过对3500余家制造业上市公司2022年年报数据的综合评估,评选出百强企业。研究显示,百强企业专注主业,半数以上成长为制造业单项冠军;民营企业在盈利效率、创新发展方面表现优异;东部地区引领发展,装备制造业领先,新能源产业呈现爆发性增长。百强企业在科技创新、质效提升、持续增长、稳定就业等方面发挥重要作用,但也存在品牌建设和创新水平差距、领军企业竞争力提升空间、高端领域龙头企业培育不足等问题。 适用人群:制造业企业管理者、政策制定者、投资者及相关研究人员。 使用场景及目标:①帮助企业管理者了解行业发展趋势,提升企业竞争力;②为政策制定者提供决策参考,推动制造业高质量发展;③为投资者提供投资参考,识别优质企业;④为研究人员提供详实数据,助力学术研究。 其他说明:报告建议从重突破促升级、重创新补短板、重质量树品牌三个方面进一步推进制造业企业高质量发展,以加快建设具有全球竞争力的一流企业。
内容概要:本文详细介绍了异步电机无感矢量控制仿真的关键技术与常见问题解决方案。首先讨论了坐标变换(Clarke和Park变换)的基础操作及其注意事项,强调了正确选择系数的重要性。接下来深入探讨了滑模观测器的设计与优化方法,包括使用查表法替代三角函数计算以提高效率,以及加入低通滤波器减少高频抖振。此外,文章还涉及了速度估算的方法,如频域法和改进型滑模观测器的应用,并提供了具体的Python和Matlab代码片段。最后,针对电流环控制提出了前馈补偿机制,确保在突加负载情况下仍能保持良好的电流跟踪效果。文中多次提到调参技巧,特别是对于PI参数的选择给出了实用建议。 适合人群:从事电机控制系统研究与开发的技术人员,尤其是对异步电机无感矢量控制感兴趣的工程师。 使用场景及目标:适用于希望深入了解并掌握异步电机无感矢量控制仿真技术的研究人员和技术开发者。主要目标是在没有编码器的情况下实现对电机转速和扭矩的精确控制,同时提供详细的代码实现指导和调试经验。 其他说明:文章不仅提供了理论知识,还包括大量实际操作中的经验和教训,帮助读者避免常见的陷阱,快速搭建起有效的仿真环境。
# 基于Arduino的火箭动力学参数监测项目 ## 项目简介 这是一个基于Arduino平台的火箭动力学参数监测项目,旨在通过Adafruit BMP280压力传感器和Adafruit LIS3DH加速度传感器收集火箭飞行过程中的环境数据和运动数据。项目结合了Adafruit的BMP280库和LIS3DH库,实现对传感器数据的读取、处理及初步分析。 ## 项目的主要特性和功能 1. 环境数据监测通过BMP280压力传感器,实时监测并记录火箭周围的气压、温度和海拔高度变化。 2. 运动数据监测借助LIS3DH加速度传感器,获取火箭在飞行过程中的加速度、速度及方向变化数据。 3. 数据处理与传输Arduino负责收集和初步处理这些数据,然后通过串行通信或其他方式将数据发送到地面站或飞行控制软件。 4. 安全与警报基于收集的数据,项目可设置警报阈值,当超过预设的安全限制时,触发警报或采取相应的安全措施。 ## 安装使用步骤
# 基于Arduino的EPSleepy智能家居控制系统 ## 一、项目简介 EPSleepy是一个基于Arduino的智能家居控制系统原型。该项目旨在通过Arduino控制ESP32 WiFi和蓝牙板,结合MP3模块、shiftregister和按钮等硬件,实现智能家居的自动化控制。 ## 二、项目的主要特性和功能 1. 自动化控制通过Arduino代码控制ESP32板,实现家居设备的自动化控制。 2. 多种硬件支持支持MP3模块、shiftregister和按钮等硬件,实现音频播放、灯光控制、SD驱动等功能。 3. 模块化设计代码采用模块化设计,方便测试每个部分的功能,方便维护和调试。 4. 图形化界面可通过按钮和LED等硬件进行图形化操作和控制。 ## 三、安装使用步骤 1. 下载并解压项目源码文件。 2. 打开Arduino IDE,导入项目代码。 3. 连接硬件,包括ESP32板、MP3模块、shiftregister和按钮等。
Delphi 12.3控件之PowerPDF for Delphi11 FullSource.zip
内容概要:本文深入探讨了中微CMS32M5533在800W角磨机方案中的应用,涵盖硬件设计和软件实现的关键技术。硬件方面,介绍了三相桥驱动电路、MOSFET选择、电流检测电阻、PCB布局等细节;软件方面,重点讲解了反电动势检测算法、ADC采样时机、PWM配置以及换相时机的动态补偿。此外,还提供了调试技巧和成本控制方法。 适合人群:从事电动工具开发的技术人员,尤其是对电机控制有一定经验的研发人员。 使用场景及目标:适用于希望深入了解电动工具控制系统的设计和优化,特别是希望通过反电动势检测减少霍尔传感器使用的开发者。目标是提高系统的可靠性和性能,同时降低成本。 其他说明:文中提供的代码片段和硬件设计细节有助于实际项目的开发和调试。建议读者结合提供的GitHub资源进行实践,并关注硬件选型和PCB布局的注意事项。
CEO的绿色经历是指该首席执行官(CEO)在其个人职业发展过程中,所积累的与环境保护、可持续发展、绿色经济等相关的教育背景、工作经验或社会活动经验。 涵盖了教育背景、工作经验、社会活动与个人价值观等多个方面。这些经历不仅塑造了CEO对环境保护和可持续发展的认知和态度,还可能影响他们在企业决策中优先考虑环保因素的程度,从而对企业的长期发展和环境保护产生重要影响。 根据现有研究(姜付秀和黄继承,2013;许年行和李哲,2016),从高管个人简历数据中查找CEO以前是否接受过“绿色”相关教育或从事过“绿色”相关工作,若企业CEO具有绿色经历,Green取值1,否则,取值0。 数据 Stkcd、年份、D0801c、Green、股票简称、行业名称、行业代码、制造业取两位代码,其他行业用大类、当年ST或PT为1,否则为0、样本区间内ST或PT为1,否则为0、金融业为1,否则为0、制造业为1,否则为0、沪深A股为1,否则为0、第一种重污染行业为1,否则为0、第二种重污染行业为1,否则为0、第三种重污染行业为1,否则为0、产权性质,国企为1,否则为0、所属省份代码、所属城市代码、所在省份、所在地级市
内容概要:本文详细介绍了利用COMSOL Multiphysics对18650电池组进行蛇形液冷系统仿真的全过程。首先探讨了快充场景下电池过热的风险及其对电动车安全性和寿命的影响。接着,通过集总电池模型简化电化学反应,重点分析了电池产热方程和温度对产热的影响。随后,深入讨论了蛇形流道几何参数优化,如流道宽度与压降之间的非线性关系,以及流固交界面处理方法。此外,还涉及了多物理场耦合求解技巧,包括流场与传热模块的设置,以及后处理阶段的数据提取和可视化。最终得出优化设计方案,显著降低了电池组的最高温度和温度不均性。 适合人群:从事电动汽车电池管理系统设计的研究人员和技术工程师,尤其是熟悉COMSOL仿真工具的专业人士。 使用场景及目标:适用于需要评估和优化电动汽车电池组热管理系统的场合,旨在提高电池组的安全性和使用寿命,同时减少能量损耗。 其他说明:文中提供了大量具体的代码片段和参数设置建议,有助于读者快速上手并应用于实际工程项目中。
内容概要:本文详细介绍了CCSDS LDPC译码器的设计与实现,主要采用了修正最小和译码算法。该算法通过对传统最小和算法的改进,引入缩放因子α,提高了译码性能。文中具体讨论了(8176,7154)和(1280,1024)两种码组的应用场景及其优劣,并展示了如何通过C语言和Vivado进行仿真和硬件实现。此外,文章还探讨了硬件实现中的关键技术,如定点化处理、校验矩阵的压缩存储、动态阈值机制以及硬件流水线设计等。 适合人群:从事通信系统开发的研究人员和技术人员,尤其是对LDPC编码和译码感兴趣的工程师。 使用场景及目标:①帮助研究人员理解和实现CCSDS LDPC译码器;②为实际工程项目提供高效的译码解决方案;③提高译码性能,减少误码率,提升通信系统的可靠性和效率。 其他说明:文章不仅提供了理论分析,还包括了大量的代码示例和实践经验分享,有助于读者全面掌握CCSDS LDPC译码器的设计与实现。
# 基于Arduino的超声波距离测量系统 ## 项目简介 本项目是一个基于Arduino平台的超声波距离测量系统。系统包含四个超声波传感器(SPS)模块,用于测量与前方不同方向物体的距离,并通过蜂鸣器(Buzz)模块根据距离范围给出不同的反应。 ## 项目的主要特性和功能 1. 超声波传感器(SPS)模块每个模块包括一个超声波传感器和一个蜂鸣器。传感器用于发送超声波并接收回波,通过计算超声波旅行时间来确定与物体的距离。 2. 蜂鸣器(Buzz)模块根据超声波传感器测量的距离,蜂鸣器会给出不同的反应,如延时发声。 3. 主控制器(Arduino)负责控制和管理所有传感器和蜂鸣器模块,通过串行通信接收和发送数据。 4. 任务管理通过主控制器(Arduino)的 loop() 函数持续执行传感器任务(Task),包括测距、数据处理和蜂鸣器反应。 ## 安装使用步骤 1. 硬件连接
主角跑步动作素材图包含6张图片
企业数字化转型是指企业或组织将传统业务转化为数字化业务,利用人工智能、大数据、云计算、区块链、5G等数字技术提升业务效率和质量的过程。 当无形资产明细项包含“软件”“网络”“客户端”“管理系统”“智能平台”等与数字化转型技术相关的关键词以及与此相关的专利时,将该明细项目界定为“数字化技术无形资产”,再对同一公司同年度多项数字化技术无形资产进行加总,计算其占本年度无形资产的比例,即为企业数字化转型程度的代理变量。 本数据包含:原始数据、参考文献、代码do文件、最终结果。 参考文献:张永珅,李小波,邢铭强-企业数字化转型与审计定价[J].审计研究,2021(03):62-71. 数据 证券代码、证券简称、统计截止日期、报表类型、无形资产净额、资产总计、年份、期末余额(元)、数字化转型。
该资源为h5py-3.1.0-cp36-cp36m-win_amd64.whl,欢迎下载使用哦!
内容概要:本文介绍了一种基于QRBayes-LSTM的多/单变量时序预测方法,适用于不确定性强的场景如股票预测和电力负荷预测。该方法结合了分位数回归和贝叶斯优化,不仅能提供未来的趋势预测,还能给出预测值的置信区间。文中详细解释了数据准备、模型结构、损失函数设计、训练配置以及预测结果的可视化和评估指标。此外,还提供了变量重要性分析的方法,帮助理解哪些特征对预测结果的影响最大。 适合人群:从事数据分析、机器学习研究的专业人士,尤其是关注时序预测和不确定性量化的人群。 使用场景及目标:① 对于需要进行时序预测并希望获得置信区间的用户;② 关注模型性能评估和变量重要性的研究人员;③ 寻求提高预测精度和可靠性的从业者。 其他说明:本文提供的代码可以直接应用于Excel格式的数据,用户只需将数据导入即可运行。需要注意的是,为了获得最佳效果,应该确保数据格式正确并且符合特定的要求。
内容概要:本文详细介绍了ADAS(高级驾驶辅助系统)中四个主要功能模块的设计与实现,分别是自适应巡航控制系统(ACC)、前向碰撞预警系统(FCW)、自动紧急制动系统(AEB)和车道保持辅助系统(LKA)。文章不仅展示了各个系统的具体算法实现,如ACC中的PID控制、FCW中的TTC计算、AEB中的状态机设计和LKA中的PD控制器,还分享了许多实际开发中的经验和挑战,如参数调校、传感器融合、时间同步等问题。此外,文中还提到了一些有趣的细节,如在暴雨天气下LKA的表现优化,以及AEB系统在测试过程中遇到的各种corner case。 适合人群:汽车电子工程师、自动驾驶研究人员、嵌入式软件开发者。 使用场景及目标:帮助读者深入了解ADAS系统的工作原理和技术细节,掌握关键算法的实现方法,提高在实际项目中的开发和调试能力。 其他说明:文章通过生动的语言和具体的代码示例,使复杂的理论变得通俗易懂,有助于初学者快速入门并深入理解ADAS系统的开发流程。
内容概要:文章主要阐述了2023年中国高端制造业上市公司的发展概况,包括行业与区域两个维度的分布详情。从行业上看,高端制造业上市公司超过2400家,其中机械制造以628家的数量位居首位,电子(352家)和电力制造(336家)紧随其后,而像航空航天国防等也有一定的占比。从区域分布来看,广东、江苏、浙江三省处于领先地位,分别有410家、342家和199家,这表明东南沿海地区对于高端制造业的发展具有显著优势。数据来源于中国上市公司协会以及Wind。 适合人群:对中国经济结构、产业发展趋势感兴趣的读者,尤其是关注高端制造业发展的投资者、政策制定者及研究人员。 使用场景及目标:①帮助投资者了解中国高端制造业上市公司的行业布局,为投资决策提供参考依据;②为政策制定者提供数据支持,助力优化产业布局和发展规划;③供研究人员分析中国高端制造业的现状与未来发展趋势。 阅读建议:本文提供了丰富的数据和图表,读者应重点关注各行业的具体数据及其背后反映出的产业特点,同时结合区域分布情况,深入理解中国高端制造业的发展格局。
# 基于Python的机器学习算法实践 ## 项目简介 本项目旨在通过实践常用机器学习算法,提高数据挖掘和推荐系统的准确性,解决信息过载问题。应用场景包括电商、新闻、视频等网站,帮助用户更高效地获取所需信息。 ## 项目的主要特性和功能 数据挖掘实现多种数据挖掘算法,帮助用户从大量数据中提取有价值的信息。 机器学习算法包括常用的分类、回归、聚类等算法,提供详细的实现和示例程序。 推荐系统通过机器学习算法提高推荐系统的准确性,优化用户体验。 ## 安装使用步骤 1. 下载源码用户已下载本项目的源码文件。 2. 安装依赖 bash pip install r requirements.txt 3. 运行示例程序 bash python main.py 4. 自定义数据根据需要替换数据文件,重新运行程序以应用新的数据。