Ultra-QuickSort
Time Limit: 7000MS | Memory Limit: 65536K | |
Total Submissions: 39782 | Accepted: 14340 |
Description
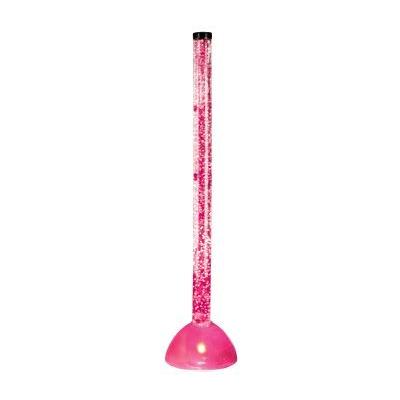
9 1 0 5 4 ,
Ultra-QuickSort produces the output
0 1 4 5 9 .
Your task is to determine how many swap operations Ultra-QuickSort needs to perform in order to sort a given input sequence.
Input
The input contains several test cases. Every test case begins with a line that contains a single integer n < 500,000 -- the length of the input sequence. Each of the the following n lines contains a single integer 0 ≤ a[i] ≤ 999,999,999, the i-th input sequence element. Input is terminated by a sequence of length n = 0. This sequence must not be processed.
Output
For every input sequence, your program prints a single line containing an integer number op, the minimum number of swap operations necessary to sort the given input sequence.
Sample Input
5 9 1 0 5 4 3 1 2 3 0
Sample Output
6 0
题意:
给出数 n (0 ~ 500000)代表有 n 个数,后给出这 n 个数(0 ~ 999999999),求出需要交换的次数使这个序列变为递增序列。
思路:
树状数组 + 离散化。离散化用 排序 + map 会导致 TLE,所以要用 排序 + 去重 + 二分 来离散化,结果应该要为 long long 型的。题目即为求逆序数,运用树状数组即可求出。
AC:
#include <cstdio> #include <cstring> #include <algorithm> using namespace std; typedef long long ll; const ll MAX = 500005; ll num[MAX], s_num[MAX]; ll bit[MAX], n; void add(ll i, ll x) { while (i <= n) { bit[i] += x; i += i & -i; } } ll sum(ll i) { ll s = 0; while (i > 0) { s += bit[i]; i -= i & -i; } return s; } int main() { while (~scanf("%I64d", &n) && n) { for (ll i = 0; i < n; ++i) { scanf("%I64d", &num[i]); s_num[i] = num[i]; bit[i + 1] = 0; } sort(s_num, s_num + n); ll ans = 0; for (ll i = 0; i < n; ++i) { int in = lower_bound(s_num, s_num + n, num[i]) - s_num; ans += i - sum(in); add(in + 1, 1); } printf("%I64d\n", ans); } return 0; }
相关推荐
【标题】"POJ2299-Ultra-QuickSort"是北京大学在线判题系统POJ上的一道编程题目,其主要涉及的算法是快速排序(Ultra-QuickSort)。快速排序是一种高效的排序算法,由C.A.R. Hoare在1960年提出。它的基本思想是采用...
主要运用合并排序合的过程,在合的过程中,判断左边是否大于右边,如果是的话,就表示有一个你序对,但是合并排序当判断左边大于右边的时候,右边的值会马上被抽出来,所以如果左边还有比右边大的数的话就判断不了了...
pku acm 2299 Ultra-QuickSort代码,合并排序求逆序数,解题报告请访问:http://blog.csdn.net/china8848
CUDA-quicksort 是一种基于 GPU 的快速排序算法实现。 CUDA-quicksort 旨在利用现代 NVIDIA GPU 的计算能力。 “文献中介绍了两种基于 GPU 的快速排序实现:GPU 快速排序,一种计算统一设备架构 (CUDA) 迭代实现,...
sort-quicksort-asc 快速排序( arr ) 使用从未排序的数字数组生成排序(升序)数组。 例子 // Direct to lib directory var qsort = require ( './../lib' ) ; // Create some data var data = new Array ( 5 ) ...
前端大厂最新面试题-quickSort
快速排序的平均时间复杂度为O(n log n),最坏情况(输入数组已排序或逆序)下为O(n^2),但这种情况很少发生,且可以通过随机化枢轴选择来避免。空间复杂度为O(log n)(递归栈的深度),是原地排序算法,不需要额外的...
QuickSort-QuickSort
public class QuickSort { public static void quickSort(int[] arr) { if (arr == null || arr.length ) { return; } quickSort(arr, 0, arr.length - 1); } public static void quickSort(int[] arr...
在C语言中,数组是一种非常基础且重要的数据结构,它允许程序员存储一组相同类型的数据。本教程将深入探讨C语言中的数组操作,特别是如何找出两个数组的交集。首先,让我们理解数组的基本概念。 数组是由相同类型的...
public class QuickSort { public static void quickSort(int[] arr) { if (arr == null || arr.length ) { return; } quickSort(arr, 0, arr.length - 1); } public static void quickSort(int[] arr...
快速排序源代码,采用C++编写,经测试未发现BUG,供大家参考
它的基本思想是分治法,通过选取一个“基准”元素,将数组分为两个子数组,使得一个子数组的所有元素都小于或等于基准,另一个子数组的所有元素都大于基准。然后对这两个子数组进行递归排序,最终合并结果。随机快速...
后缀数组(Suffix Array)是一种数据结构,用于高效地存储和检索字符串的后缀。它在文本处理、生物信息学和搜索引擎等领域有广泛的应用。DSA-IS(Disk-based Suffix Array - In-place Sorting)是针对超大数据量的...
快速排序的时间复杂度在平均情况下是O(n log n),最坏情况(如已排序数组)是O(n^2),但这种情况在实际应用中很少发生,因为可以通过随机化基准元素的选择来避免。空间复杂度主要取决于递归深度,一般为O(log n)。 ...
TIA博途是西门子公司推出的自动化工程集成软件,其中的快速排序算法FB全局库是该软件中用于实现快速排序功能的函数块库。快速排序是一种高效的排序算法,采用分治法的策略,其基本思想是:先从数列中选取一个数作为...
基础算法和数据结构:DSA 可视化算法:视觉算法 C ++面试:Huihut采访 BTree实现:B-Tree C ++对象模型线段树:SegmentTree C ++面试总结(多方面) C#面试总结(基础) 线段树:SegmentTree 算法 种类 QuickSort ...
其核心思想是选择一个"枢轴"(pivot)元素,然后将数组分为两部分:一部分包含小于枢轴的元素,另一部分包含大于枢轴的元素。递归地对这两部分继续执行同样的操作,直到数组完全有序。快速排序的时间复杂度为O(nlogn...
在这个项目"Quickselect-QuickSort-with-Covid19Data"中,作者将这两种算法应用于处理Covid19的数据,这可能是为了进行数据分析或可视化,以理解全球疫情的发展趋势。 首先,让我们深入了解一下快速排序(QuickSort...
冒泡排序是一种基础的排序算法,它通过重复遍历待排序的数组,比较相邻元素并交换位置,使得每个遍历过程都将当前未排序的最大(或最小)元素“冒泡”到数组的末尾,直到整个数组有序。在Java中实现冒泡排序,我们...