In Spring 3, one of the feature of “mvc:annotation-driven“, is support for convert object to/from XML file, if JAXB is in project classpath.
In this tutorial, we show you how to convert a return object into XML format and return it back to user via Spring @MVC framework.
Technologies used :
- Spring 3.0.5.RELEASE
- JDK 1.6
- Eclipse 3.6
- Maven 3
JAXB is included in JDK6, so, you do not need to include JAXB library manually, as long as object is annotated with JAXB annotation, Spring will convert it into XML format automatically.
1. Project Dependencies
No extra dependencies, you need to include Spring MVC in your Maven pom.xml
only.
<properties> <spring.version>3.0.5.RELEASE</spring.version> </properties> <dependencies> <!-- Spring 3 dependencies --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-core</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-web</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-webmvc</artifactId> <version>${spring.version}</version> </dependency> </dependencies>
2. Model + JAXB
A simple POJO model and annotated with JAXB annotation, later convert this object into XML output.
package com.mkyong.common.model; import javax.xml.bind.annotation.XmlElement; import javax.xml.bind.annotation.XmlRootElement; @XmlRootElement(name = "coffee") public class Coffee { String name; int quanlity; public String getName() { return name; } @XmlElement public void setName(String name) { this.name = name; } public int getQuanlity() { return quanlity; } @XmlElement public void setQuanlity(int quanlity) { this.quanlity = quanlity; } public Coffee(String name, int quanlity) { this.name = name; this.quanlity = quanlity; } public Coffee() { } }
3. Controller
Add “@ResponseBody” in the method return value, no much detail in the Spring documentation.
As i know, when Spring see
- Object annotated with JAXB
- JAXB library existed in classpath
- “mvc:annotation-driven” is enabled
- Return method annotated with @ResponseBody
It will handle the conversion automatically.
package com.mkyong.common.controller; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import org.springframework.web.bind.annotation.ResponseBody; import com.mkyong.common.model.Coffee; @Controller @RequestMapping("/coffee") public class XMLController { @RequestMapping(value="{name}", method = RequestMethod.GET) public @ResponseBody Coffee getCoffeeInXML(@PathVariable String name) { Coffee coffee = new Coffee(name, 100); return coffee; } }
4. mvc:annotation-driven
In one of your Spring configuration XML file, enable “mvc:annotation-driven
“.
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:context="http://www.springframework.org/schema/context" xmlns:mvc="http://www.springframework.org/schema/mvc" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation=" http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.0.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-3.0.xsd"> <context:component-scan base-package="com.mkyong.common.controller" /> <mvc:annotation-driven /> </beans>
Alternatively, you can declares “spring-oxm.jar” dependency and include following
MarshallingView
, to handle the conversion. With this method, you don’t need annotate @ResponseBody in your method.
<beans ...> <bean class="org.springframework.web.servlet.view.BeanNameViewResolver" /> <bean id="xmlViewer" class="org.springframework.web.servlet.view.xml.MarshallingView"> <constructor-arg> <bean class="org.springframework.oxm.jaxb.Jaxb2Marshaller"> <property name="classesToBeBound"> <list> <value>com.mkyong.common.model.Coffee</value> </list> </property> </bean> </constructor-arg> </bean> </beans>
5. Demo
URL : http://localhost:8080/SpringMVC/rest/coffee/arabica
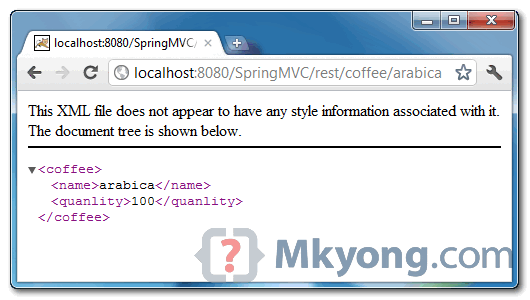
相关推荐
这个入门教程将涵盖XML配置和注解配置两种方式,帮助初学者理解如何在Spring MVC中搭建基本的"Hello, World!"应用。 首先,我们从XML配置开始。在Spring MVC中,`DispatcherServlet`是入口点,它负责分发HTTP请求到...
下面将详细介绍Spring AOP的注解方式和XML配置方式。 ### 注解方式 #### 1. 定义切面(Aspect) 在Spring AOP中,切面是包含多个通知(advisors)的类。使用`@Aspect`注解标记切面类,例如: ```java @Aspect ...
在本文中,我们将深入探讨Spring 3 MVC框架中的ContentNegotiatingViewResolver组件,这是一个用于内容协商的关键工具,它使得应用程序能够根据用户代理或请求参数返回适当格式的响应。...
Spring3MVC真正入门资料 本文将详细介绍 Spring3MVC 框架的入门知识,包括核心类与接口、核心流程图、DispatcherServlet 说明等。 核心类与接口 在 Spring3MVC 框架中,有几个重要的接口与类,了解它们的作用可以...
掌握这些知识点后,你将能够创建基本的Spring应用,并开始探索更高级的特性,如AOP、Spring MVC等。记得实践是检验真理的唯一标准,尝试自己动手编写XML配置文件并运行Spring应用,这将有助于巩固理论知识。
为了正确配置Spring3MVC,首先需要在`web.xml`文件中配置DispatcherServlet。下面是一个简单的配置示例: ```xml <servlet-name>example <servlet-class>org.springframework.web.servlet.DispatcherServlet ...
Spring 3.0 MVC的核心组件是`DispatcherServlet`,需要在`web.xml`中进行配置: ```xml <servlet-name>example <servlet-class>org.springframework.web.servlet.DispatcherServlet <load-on-startup>1 ...
在Spring 3 MVC的配置方面,我们需要创建一个servlet配置文件(如`spring-servlet.xml`),其中定义了Bean、视图解析器和其他相关组件。例如,我们可以定义一个Controller Bean,如下所示: ```xml ...
3. **servlet-context.xml**:Spring MVC的配置文件。 ```xml <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context=...
在IT行业中,MyBatis和Spring3 MVC是两个非常重要的框架,它们经常被结合使用来构建高效、可维护的Web应用程序。本项目标题为“mybatis+spring3 mvc 登录”,这表明我们将探讨如何利用这两个框架实现一个基础的用户...
2. **Spring配置**:在src/main/resources下,可能有Spring的配置文件如`applicationContext.xml`或`dispatcher-servlet.xml`,定义了bean的实例化、依赖注入以及Spring MVC的相关配置。 3. **Controller**:Spring...
- **spring-mvc-config.xml**: Spring MVC的核心配置文件,包括Bean定义、HandlerMapping和ViewResolver等。 ```xml <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi=...
XML配置是Spring MVC早期版本中常见的配置方式,它允许开发者通过XML文件来定义组件、映射URL和设置拦截器等。在本篇文章中,我们将深入探讨如何在Spring MVC中使用XML配置来实现拦截器。 拦截器(Interceptor)在...
Spring3 MVC 是一款基于Java的轻量级Web应用框架,它是Spring框架的重要组成部分,主要用于构建Web应用程序的模型-视图-控制器(MVC)结构。这个"最新Spring3 MVC 示例 demo程序"旨在帮助开发者理解并掌握Spring 3的...
以上内容详细介绍了`spring+mvc+mybatis`在IDEA中的XML配置,包括Spring的bean管理、Spring MVC的Web处理以及MyBatis的数据访问。理解并正确配置这些XML文件对于开发者来说至关重要,因为它们是构建高效、可维护的...
为了使 Spring MVC 能够处理这个请求,我们需要在 `web.xml` 文件中配置一个 Servlet。 ```xml <?xml version="1.0" encoding="ISO-8859-1"?> <web-app xmlns="http://java.sun.com/xml/ns/j2ee" xmlns:xsi=...
**Spring MVC XML详解** Spring MVC 是 Spring 框架的一部分,专门用于构建Web应用程序。它提供了模型-视图-控制器(MVC)架构,帮助开发者有效地分离业务逻辑、数据处理和用户界面。在这个主题中,我们将深入探讨...
3. **生成服务端点**:使用Spring的`WebServiceGatewaySupport`或`WebServiceTemplate`类作为基础,创建一个服务处理器类,该类将处理来自客户端的请求,并调用业务逻辑。 4. **配置Spring MVC**:在Spring MVC的...
本篇文章将详细解析在Java环境下快速搭建Spring MVC所需的关键配置文件,包括`web.xml`、`spring-mvc.xml`、`spring-service.xml`、`spring-dao.xml`以及*mapper.xml文件。 首先,`web.xml`是Java Web应用的部署...
Spring3MVC-REST-HelloWorld 是一个基础的示例,用于展示如何在Spring框架的MVC模块中实现RESTful Web服务。这个实例是初学者理解Spring MVC与REST结合使用的理想起点。REST(Representational State Transfer)是一...