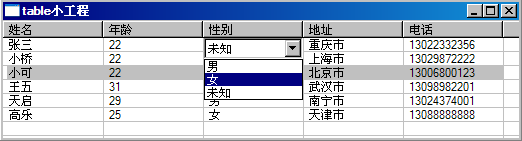
package cn.zcq100.Demo1;
import javax.swing.text.TabExpander;
import org.eclipse.swt.SWT;
import org.eclipse.swt.custom.TableEditor;
import org.eclipse.swt.events.ModifyEvent;
import org.eclipse.swt.events.ModifyListener;
import org.eclipse.swt.events.MouseAdapter;
import org.eclipse.swt.events.MouseEvent;
import org.eclipse.swt.events.SelectionAdapter;
import org.eclipse.swt.events.SelectionEvent;
import org.eclipse.swt.graphics.Point;
import org.eclipse.swt.graphics.Rectangle;
import org.eclipse.swt.layout.FillLayout;
import org.eclipse.swt.widgets.Combo;
import org.eclipse.swt.widgets.Control;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Event;
import org.eclipse.swt.widgets.Listener;
import org.eclipse.swt.widgets.Menu;
import org.eclipse.swt.widgets.MenuItem;
import org.eclipse.swt.widgets.MessageBox;
import org.eclipse.swt.widgets.Shell;
import org.eclipse.swt.widgets.Spinner;
import org.eclipse.swt.widgets.Table;
import org.eclipse.swt.widgets.TableColumn;
import org.eclipse.swt.widgets.TableItem;
import org.eclipse.swt.widgets.Text;
public class TableDemo {
private TableEditor editor = null;
private Table table = null;
public static void main(String[] args) {
new TableDemo();
}
private TableDemo() {
Display display = new Display();
Shell shell = new Shell(display);
shell.setLayout(new FillLayout());
shell.setText("table小工程");
createTable(shell);
shell.pack();
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch())
display.sleep();
}
display.dispose();
}
/**
* 创建表格
*
* @param shell
*/
private void createTable(final Shell shell) {
table = new Table(shell, SWT.MULTI | SWT.FULL_SELECTION);
editor = new TableEditor(table);
editor.horizontalAlignment = SWT.LEFT;
editor.grabHorizontal = true;
table.setHeaderVisible(true);
table.setLinesVisible(true);
TableColumn col1 = new TableColumn(table, SWT.LEFT);
col1.setText("姓名");
col1.setWidth(100);
TableColumn col2 = new TableColumn(table, SWT.LEFT);
col2.setText("年龄");
col2.setWidth(100);
TableColumn col5 = new TableColumn(table, SWT.LEFT);
col5.setText("性别");
col5.setWidth(100);
TableColumn col3 = new TableColumn(table, SWT.LEFT);
col3.setText("地址");
col3.setWidth(100);
TableColumn col4 = new TableColumn(table, SWT.LEFT);
col4.setText("电话");
col4.setWidth(100);
/**
* 添加表格数据
*/
new TableItem(table, SWT.LEFT).setText(new String[] { "张三", "22", "男",
"重庆市", "13022332356" });
new TableItem(table, SWT.LEFT).setText(new String[] { "小桥", "22", "女",
"上海市", "13029872222" });
new TableItem(table, SWT.LEFT).setText(new String[] { "小可", "22", "男",
"北京市", "13006800123" });
new TableItem(table, SWT.LEFT).setText(new String[] { "王五", "31", "男",
"武汉市", "13098982201" });
new TableItem(table, SWT.LEFT).setText(new String[] { "天启", "29", "男",
"南宁市", "13024374001" });
new TableItem(table, SWT.LEFT).setText(new String[] { "高乐", "25", "女",
"天津市", "13088888888" });
// 删除菜单
Menu menu1 = new Menu(shell, SWT.POP_UP);
table.setMenu(menu1);
MenuItem menuitem1 = new MenuItem(menu1, SWT.PUSH);
menuitem1.setText("删除");
menuitem1.addListener(SWT.Selection, new Listener() {
@Override
public void handleEvent(Event event) {
MessageBox mbox = new MessageBox(shell, SWT.DIALOG_TRIM|SWT.ICON_INFORMATION);
mbox.setText("删除成功");
mbox.setMessage("删除了" + table.getSelectionCount() + "条记录");
table.remove(table.getSelectionIndices());
mbox.open();
}
});
// 修改table
{
table.addMouseListener(new MouseAdapter() {
@Override
public void mouseDoubleClick(MouseEvent e) {
Control c = editor.getEditor();
if (c != null) {
c.dispose();
}
// 得到选中的行
Point point = new Point(e.x, e.y);
// MessageDialog.openInformation(shell, null,
// point.x+","+point.y);
final TableItem tableitem = table.getItem(point);
// 得到选中的列
int column = -1;
for (int i = 0; i < table.getColumnCount(); i++) {
Rectangle rec = tableitem.getBounds(i);
if (rec.contains(point))
column = i;
}
final int col1 = column;
//修改年龄,进行微调
if(col1==1){
final Spinner spiner=new Spinner(table,SWT.NONE);
spiner.setMaximum(120);
spiner.setMinimum(1);
spiner.setSelection(new Integer(tableitem.getText(1)));
editor.setEditor(spiner, tableitem, col1);
spiner.addModifyListener(new ModifyListener() {
@Override
public void modifyText(ModifyEvent e) {
tableitem.setText(col1,String.valueOf(spiner.getSelection()));
}
});
}else if (col1 == 2) {
//修改性别用下拉列表
final Combo comb = new Combo(table, SWT.READ_ONLY);
comb.setItems(new String[] { "男", "女", "未知" });
comb.addSelectionListener(new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent e) {
tableitem.setText(col1, comb.getText());
comb.dispose();
super.widgetSelected(e);
}
});
editor.setEditor(comb, tableitem, column);
}else{
//其他的修改都是用文本框
final Text txt=new Text(table,SWT.NONE);
txt.setText(tableitem.getText(col1));
txt.forceFocus();
editor.setEditor(txt, tableitem, col1);
txt.addModifyListener(new ModifyListener() {
@Override
public void modifyText(ModifyEvent e) {
tableitem.setText(col1, txt.getText());
}
});
}
}
});
}
}
}
分享到:
相关推荐
本文将深入探讨SWT `Table`控件中的单元格编辑功能,并通过实例代码进行详细解析。 #### 1. 创建TableEditor `TableEditor`是SWT提供的一种编辑器,专门用于处理`Table`中的单元格编辑。在创建`TableEditor`时,需...
标题"SWT tableEditor删除后刷新"涉及的问题可能是关于在删除TableEditor实例后,如何正确地更新或刷新表格显示。在使用TableEditor时,我们可能遇到的情况是,当编辑器被创建并应用到一个单元格后,如果用户进行了...
TableColumn column1 = new TableColumn(tree, SWT.LEFT); column1.setText("Column 1"); // 添加更多列... ``` 4. **数据模型和内容提供者** - 创建一个数据模型,通常是一个对象列表,代表树的各个节点。 - ...
创建一个Table,你需要实例化`org.eclipse.swt.widgets.Table`类,并将其添加到父容器中。你可以通过调用`TableColumn`类的方法来添加列,设置列头文本,调整列宽。`TableItem`用于创建和管理表中的行数据。 然而,...
1. **基础控件的使用**:如何创建和配置SWT和JFace的基本组件,如Button、Text、Table等。 2. **布局管理**:讲解如何使用GridLayout、FormLayout、FillLayout等布局管理器来组织控件。 3. **事件处理**:如何编写...
1. **控件使用**:SWT包含各种常见的GUI控件,如按钮(Button)、文本框(Text)、列表(List)、树形视图(Tree)、表格(Table)等。通过实例演示,你可以了解这些控件的创建、布局和事件处理。 2. **布局管理**...
**KTable for SWT 源代码与实例解析** 在Java的SWT(Standard Widget Toolkit)框架下,开发人员经常寻找适合展示大量数据的组件。KTable是一个强大的、可定制的表格控件,专为SWT设计,提供了丰富的功能,如排序、...
SWT 提供了一系列的组件,如按钮(Button)、文本框(Text)、标签(Label)、列表(List)、树(Tree)、表格(Table)等,用于构建用户界面。这些组件可以通过继承自`org.eclipse.swt.widgets.Widget`类的子类来...
6. **表和树**:SWT 和 JFace 支持复杂的数据展示,如表格(Table)和树形结构(Tree)。通过实例,我们可以学习如何加载数据、设置列、添加排序和过滤功能,以及处理用户的选择事件。 7. **事件处理**:事件驱动是...
Table table = new Table(parent, SWT.MULTI | SWT.FULL_SELECTION); TableViewer tableViewer = new TableViewer(table); ``` 2. **定义表格列** 使用`TableViewerColumn`来定义表格的列,可以设置列头文本、宽度...
本资源包含的"Sample Code"可能是各种基于SWT的实例代码,这些代码涵盖了SWT的各种组件、布局管理、事件处理、对话框、拖放操作、打印、剪贴板支持等高级主题。通过这些源码,我们可以学习到以下关键知识点: 1. **...
开发者可以方便地实例化`TableManager`,并通过其提供的API来操作表格,无需关心底层的复杂实现。 总之,`SWT表格管理类`通过`TableManager`这样的辅助工具,使得在Java环境中创建功能强大的表格变得简单易行。通过...
5. **Table**: 创建表格控件,如`Table table = new Table(shell, SWT.MULTI | SWT.H_SCROLL | SWT.V_SCROLL);` ### SWT事件处理 - 使用`addSelectionListener`添加选择事件监听器。 - `Listener`接口提供了`...
SWT实例编程 SWT提供了丰富的UI组件,以下是一些常用组件的简要介绍: - **Button组件**:用于创建按钮,可以通过点击触发事件。 - **Label组件**:用于显示静态文本或图像。 - **Text组件**:用于输入和编辑文本...
`TableEditor` 类被实例化,它将被用来在 `Table` 的单元格上显示编辑器控件。我们设置了编辑器的一些属性,例如水平对齐方式、是否自动扩展宽度以及最小宽度。 关键的部分在于 `Table` 的 `addSelectionListener` ...
- Table:SWT 提供的 `Table` 类可以用来创建表格,支持多列、排序、选择等特性。JFace 的 `TableViewer` 可以进一步增强表格功能,如数据绑定和行操作。 - Tree:`Tree` 组件用于展示层次化的数据结构,`...
SWT实例编程 SWT提供了广泛的组件供开发者选择。下面是一些常见的组件示例: - **Button组件**:用于创建按钮,可以响应用户的点击事件。 - **Label类组件**:用于显示静态文本或图标。 - **Text组件**:用于输入...
Table table = new Table(shell, SWT.BORDER | SWT.FULL_SELECTION); table.setHeaderVisible(true); table.setLinesVisible(true); ``` 6. **List**:用于显示列表项。 - 创建方式: ```java List list = ...
2. **扩展组件**:除了基本组件,SWT还提供了一些更复杂的组件,如树形视图(Tree),表格(Table),进度条(ProgressBar),滑块(Slider)等。这些组件增强了用户界面的交互性和信息展示能力。例如,`Tree`用于...
本教程将通过一个在Eclipse环境下实现的SWT实例,帮助你理解和掌握如何利用SWT创建图形化界面。 首先,我们需要了解SWT的基本元素。SWT包含了一系列的控件,如按钮(Button)、文本框(Text)、标签(Label)、表格...