转于:http://my.oschina.net/u/861587/blog/105605
-------------------------------------------------------------------------------------------
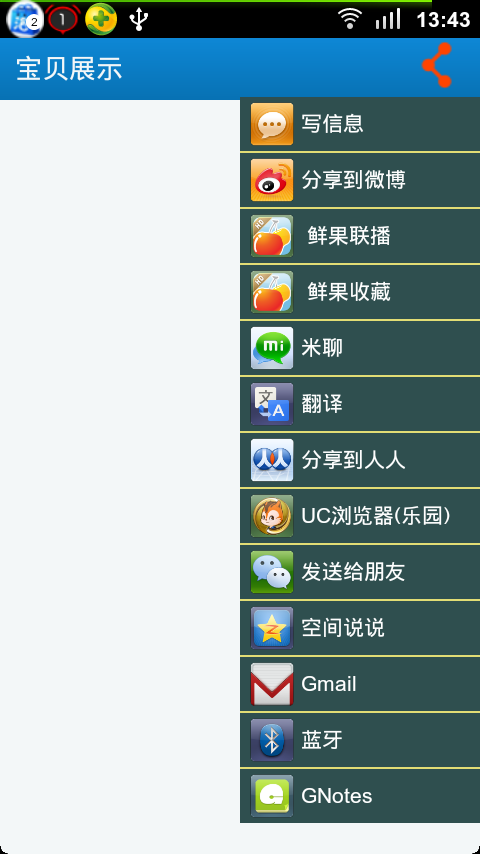
1、布局:
popup_share.xml
01 |
<?xml version= "1.0" encoding= "utf-8" ?>
|
02 |
<LinearLayout xmlns:android= "http://schemas.android.com/apk/res/android" |
03 |
android:layout_width= "fill_parent" |
04 |
android:layout_height= "wrap_content" >
|
06 |
android:id= "@+id/share_list" |
07 |
android:background= "#2F4F4F" |
08 |
android:fadingEdge= "none" |
09 |
android:layout_width= "fill_parent" |
10 |
android:layout_height= "wrap_content" |
11 |
android:cacheColorHint= "#00000000" |
12 |
android:divider= "#E2DD75" |
13 |
android:dividerHeight= "1.0dip" |
14 |
android:headerDividersEnabled= "true" |
15 |
android:footerDividersEnabled= "false" />
|
popup_share_item.xml
01 |
<?xml version= "1.0" encoding= "utf-8" ?>
|
02 |
<LinearLayout xmlns:android= "http://schemas.android.com/apk/res/android" |
03 |
android:gravity= "center_vertical" |
04 |
android:layout_width= "wrap_content" |
05 |
android:layout_height= "wrap_content" |
06 |
android:padding= "2.0dip" >
|
08 |
android:id= "@+id/share_item_icon" |
09 |
android:layout_width= "32.0dip" |
10 |
android:layout_height= "32.0dip" |
11 |
android:layout_marginLeft= "3.0dip" |
12 |
android:scaleType= "fitXY" />
|
14 |
android:id= "@+id/share_item_name" |
15 |
android:gravity= "center" |
16 |
android:layout_width= "wrap_content" |
17 |
android:layout_height= "wrap_content" |
19 |
android:textColor= "@color/white" |
20 |
android:singleLine= "true" |
21 |
android:textSize= "@dimen/s_size" |
22 |
android:layout_marginLeft= "3.0dip" |
23 |
android:layout_marginRight= "3.0dip" />
|
2、查询手机内所有支持分享的应用列表
01 |
public List<ResolveInfo> getShareApps(Context context) {
|
02 |
List<ResolveInfo> mApps = new ArrayList<ResolveInfo>();
|
03 |
Intent intent = new Intent(Intent.ACTION_SEND, null );
|
04 |
intent.addCategory(Intent.CATEGORY_DEFAULT);
|
05 |
intent.setType( "text/plain" );
|
07 |
PackageManager pManager = context.getPackageManager();
|
08 |
mApps = pManager.queryIntentActivities(intent,
|
09 |
PackageManager.COMPONENT_ENABLED_STATE_DEFAULT);
|
注:ApplicationInfo是从一个特定的应用得到的信息。这些信息是从相对应的Androdimanifest.xml的< application>标签中收集到的。
ResolveInfo这个类是通过解析一个与IntentFilter相对应的intent得到的信息。它部分地对应于从AndroidManifest.xml的< intent>标签收集到的信息。
得到List列表,我自建的AppInfo类,自己建一个就行
01 |
private List<AppInfo> getShareAppList() {
|
02 |
List<AppInfo> shareAppInfos = new ArrayList<AppInfo>();
|
03 |
PackageManager packageManager = getPackageManager();
|
04 |
List<ResolveInfo> resolveInfos = getShareApps(mContext);
|
05 |
if ( null == resolveInfos) {
|
08 |
for (ResolveInfo resolveInfo : resolveInfos) {
|
09 |
AppInfo appInfo = new AppInfo();
|
10 |
appInfo.setAppPkgName(resolveInfo.activityInfo.packageName);
|
12 |
appInfo.setAppLauncherClassName(resolveInfo.activityInfo.name);
|
13 |
appInfo.setAppName(resolveInfo.loadLabel(packageManager).toString());
|
14 |
appInfo.setAppIcon(resolveInfo.loadIcon(packageManager));
|
15 |
shareAppInfos.add(appInfo);
|
3、弹出PopupWindow的实现
01 |
private void initSharePopupWindow(View parent) {
|
02 |
PopupWindow sharePopupWindow = null ;
|
04 |
ListView shareList = null ;
|
05 |
if ( null == sharePopupWindow) {
|
07 |
view = LayoutInflater.from(DetailExchangeActivity. this ).inflate(R.layout.popup_share, null );
|
08 |
shareList = (ListView) view.findViewById(R.id.share_list);
|
09 |
List<AppInfo> shareAppInfos = getShareAppList();
|
10 |
final ShareCustomAdapter adapter = new ShareCustomAdapter(mContext, shareAppInfos);
|
11 |
shareList.setAdapter(adapter);
|
13 |
shareList.setOnItemClickListener( new OnItemClickListener() {
|
16 |
public void onItemClick(AdapterView<?> parent, View view,
|
17 |
int position, long id) {
|
19 |
Intent shareIntent = new Intent(Intent.ACTION_SEND);
|
20 |
AppInfo appInfo = (AppInfo) adapter.getItem(position);
|
21 |
shareIntent.setComponent( new ComponentName(appInfo.getAppPkgName(), appInfo.getAppLauncherClassName()));
|
22 |
shareIntent.setType( "text/plain" );
|
25 |
shareIntent.putExtra(Intent.EXTRA_TEXT, "测试,这里发送推广地址" );
|
26 |
shareIntent.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
|
27 |
DetailExchangeActivity. this .startActivity(shareIntent);
|
31 |
sharePopupWindow = new PopupWindow(view,
|
32 |
( int )( 160 * density), LinearLayout.LayoutParams.WRAP_CONTENT);
|
35 |
sharePopupWindow.setFocusable( true );
|
37 |
sharePopupWindow.setOutsideTouchable( true );
|
39 |
sharePopupWindow.setBackgroundDrawable( new BitmapDrawable());
|
42 |
sharePopupWindow.showAsDropDown(parent, - 5 , 5 );
|
分享到:
相关推荐
本教程将详细介绍如何在Android中自定义ACTION_SEND功能,创建一个符合自己应用风格的分享列表。 首先,我们需要为分享列表创建相应的布局文件。`popup_share.xml`是整个分享列表的容器,通常是一个PopupWindow,...
Android 使用 Intent.ACTION_SEND 分享图片和文字内容的示例代码详解 Android mobile 操作系统中,Intent.ACTION_SEND 是一个非常常用的 Action,主要用于实现分享功能,例如分享图片、文字内容等。在本文中,我们...
分享功能是app中特别常见的功能,国内的app基本都支持分享到微信 QQ等... Intent share = new Intent(Intent.ACTION_SEND); share.setType(text/plain); share.putExtra(Intent.EXTRA_TEXT, shareText); share.pu
如果需要分享多个数据项,可以使用ACTION_SEND_MULTIPLE,同时提供一个包含所有URI的List: ```java ArrayList<Uri> uris = new ArrayList(); uris.add(uri1); uris.add(uri2); // 添加更多... shareIntent....
以上就是实现Android自定义分享列表的基本流程和注意事项。在实际开发中,可能还需要处理各种异常情况,如没有可分享应用、权限问题等。通过理解和运用这些知识点,你可以创建一个高效且用户友好的自定义分享功能。
在事件处理函数中,使用`Intent`的`ACTION_SEND`操作,并指定分享的内容类型(如文本、图片、链接等),然后使用`putExtra()`方法添加要分享的具体内容。 4. **选择分享应用** Android系统会根据`Intent`的类型...
6. ACTION_SEND: 分享内容,可以用来分享文本、图片、视频等。 7. ACTION_BROWSABLE: 表示可以被浏览器启动,通常用于Web链接。 8. ACTION_DIAL: 打开拨号界面,不需要拨打电话,只显示拨号盘。 9. ACTION_SEARCH...
Android系统内置了许多预定义的ACTION,比如ACTION_VIEW、ACTION_CALL、ACTION_SEND等,开发者也可以自定义ACTION以满足特定需求。以下是一些常见的ACTION及其用途: 1. ACTION_MAIN:这是所有应用启动的入口点,...
这些预定义的Action对应了不同的功能,开发者可以根据需要自定义Action。 ACTION_VIEW是最常见的Action之一,用于打开或显示数据,比如查看网页、图片或者视频。ACTION_CALL则用于拨打电话,ACTION_SEND则用于分享...
- 自定义Action:开发者可以定义自己的Action,以实现特定功能。 2. **Data/Type**: - 使用Uri来表示数据,例如`Uri.parse("http://www.google.com")`表示一个HTTP链接。 - 数据类型由scheme决定,如http对应...
3. **MMS权限**: 除了`SEND_SMS`,发送MMS还需要`ACCESS_FINE_LOCATION`(用于获取网络状态)和`INTERNET`权限。 4. **MMS监听**: 监听MMS消息的接收与短信类似,但广播接收器需要监听`INTENT_ACTION_WAP_PUSH_...
总结,Android调用系统分享功能的核心在于创建和启动一个ACTION_SEND的Intent。开发者可以根据不同的数据类型设置Intent,然后通过系统选择器或自定义菜单提供给用户多种分享途径。在实践中,理解和掌握这部分内容...
在Android系统中,开发者通常会通过`Intent`来实现分享功能,可以创建一个`Intent`,指定其类型为`ACTION_SEND`,然后填充要分享的内容,如文本、图片或视频等。接着,使用`Intent.createChooser()`方法创建一个分享...
在这个系统中,关键的技术点包括自定义相册选择、自定义分享列表以及多图分享功能。下面我们将深入探讨这些核心知识点。 1. 自定义相册选择: 在Android中,获取用户的照片通常需要访问设备的媒体库。原生的Intent...
常见的Action有ACTION_VIEW、ACTION_CALL、ACTION_SEND等。例如,ACTION_VIEW用于打开一个网页或文件,ACTION_CALL用于拨打电话。 3. **Data Uri** Data Uri用于携带数据,可以是URL、URI或者MIME类型,它帮助我们...
对于分享多个文件(如多张图片或多个视频),可以使用`Intent.ACTION_SEND_MULTIPLE`动作,并提供一个包含所有文件URI的数组。 ```java ArrayList<Uri> imageUris = new ArrayList(); imageUris.add(Uri.parse(...
6. **自定义分享对话框**:在`ShareDialogDemo`中,可能会有一个自定义的分享对话框(ShareDialog)来展示可供用户选择的分享应用列表。这个对话框通常会在用户点击分享按钮时弹出,显示已安装的可接收ACTION_SEND ...