题目来源:http://poj.org/problem?id=1107
Description
Weird
Wally's Wireless Widgets, Inc. manufactures an eclectic assortment of
small, wireless, network capable devices, ranging from dog collars, to
pencils, to fishing bobbers. All these devices have very small memories.
Encryption algorithms like Rijndael, the candidate for the Advanced
Encryption Standard (AES) are demonstrably secure but they don't fit in
such a tiny memory. In order to provide some security for transmissions
to and from the devices, WWWW uses the following algorithm, which you
are to implement.
Encrypting a message requires three integer keys, k1, k2, and k3.
The letters [a-i] form one group, [j-r] a second group, and everything
else ([s-z] and underscore) the third group. Within each group the
letters are rotated left by ki positions in the message. Each group is
rotated independently of the other two. Decrypting the message means
doing a right rotation by ki positions within each group.
Consider the message the_quick_brown_fox encrypted with ki values of
2, 3 and 1. The encrypted string is _icuo_bfnwhoq_kxert. The figure
below shows the decrypting right rotations for one character in each of
the three character groups.
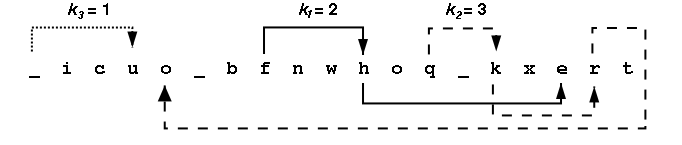
Looking at all the letters in the group [a-i] we see {i,c,b,f,h,e}
appear at positions {2,3,7,8,11,17} within the encrypted message. After a
right rotation of k1=2, these positions contain the letters
{h,e,i,c,b,f}. The table below shows the intermediate strings that come
from doing all the rotations in the first group, then all rotations in
the second group, then all the rotations in the third group. Rotating
letters in one group will not change any letters in any of the other
groups.

All input strings contain only lowercase letters and underscores(_).
Each string will be at most 80 characters long. The ki are all positive
integers in the range 1-100.
Input
Input
consists of information for one or more encrypted messages. Each problem
begins with one line containing k1, k2, and k3 followed by a line
containing the encrypted message. The end of the input is signalled by a
line with all key values of 0.
Output
For each encrypted message, the output is a single line containing the decrypted string.
Sample Input
2 3 1
_icuo_bfnwhoq_kxert
1 1 1
bcalmkyzx
3 7 4
wcb_mxfep_dorul_eov_qtkrhe_ozany_dgtoh_u_eji
2 4 3
cjvdksaltbmu
0 0 0
Sample Output
the_quick_brown_fox
abcklmxyz
the_quick_brown_fox_jumped_over_the_lazy_dog
ajsbktcludmv
此题题意是将26个字母和"_"分成3段,a~i为第一段,j~r为第二段,其他的为第三段。拿第一组测试数据来说,分为3组分别为:
t1{i c b f h e}
t2{o n o q k r}
t3{_ u _ w _ x t}
k值分别为k1=2,k2=3, k3=1,t1,t2,t3分别向右旋转ki布后得到的新数据分别为:
t1'{h e i c b f}
t2'{q k r o n o}
t3'{t _ u _ w _ x }
将跟换后的新数据替换掉在原字符串的位置即可
#include <stdio.h>
#include <string.h>
void rotate(char str[], int len, int k)
{
//将字符串向右旋转k个单位
int i, j;
char str1[81], str2[81];
k = len-k%len;
for(i = 0; i < k; i++)
{
str1[i] = str[i];
}
str1[i] = '\0';
j = 0;
for(i = k; i < len; i++)
{
str2[j++] = str[i];
}
str2[j] = '\0';
strcat(str2, str1);
strcpy(str, str2);
}
int main()
{
int i;
int k1, k2, k3;
int len1, len2, len3, len;
int n1[81], n2[81], n3[81];
char t1[81], t2[81], t3[81], str[81];
while(1)
{
scanf("%d %d %d", &k1, &k2, &k3);
if((k1+k2+k3) == 0) break;
scanf("%s", str);
len = strlen(str);
len1 = len2 = len3 = 0;
memset(t1, '\0', sizeof(t1));
memset(t2, '\0', sizeof(t2));
memset(t3, '\0', sizeof(t3));
for(i = 0; i < len; i++)
{
if(str[i] >= 'a' && str[i] <= 'i')
{
t1[len1] = str[i];
n1[len1++] = i;
}
else if(str[i] >= 'j' && str[i] <= 'r')
{
t2[len2] = str[i];
n2[len2++] = i;
}
else
{
t3[len3] = str[i];
n3[len3++] = i;
}
}
if(len1) rotate(t1, len1, k1);
if(len2) rotate(t2, len2, k2);
if(len3) rotate(t3, len3, k3);
for(i = 0; i < len1; i++)
{
str[n1[i]] = t1[i];
}
for(i = 0; i < len2; i++)
{
str[n2[i]] = t2[i];
}
for(i = 0; i < len3; i++)
{
str[n3[i]] = t3[i];
}
printf("%s\n", str);
}
return 0;
}
分享到:
相关推荐
【压缩包子文件的文件名称列表】"POJ2159-Ancient Cipher.cpp"、"POJ2159-Ancient Cipher.doc" 这两个文件很可能是解题过程中的关键文档。"POJ2159-Ancient Cipher.cpp"很可能是使用C++编程语言编写的解决该问题的...
poj-1002源码,没有题解,题解看博客poj-1002源码,没有题解,题解看博客poj-1002源码,没有题解,题解看博客poj-1002源码,没有题解,题解看博客
【标题】"POJ题解及题目分类"涵盖了ACM竞赛中的编程问题,主要使用C/C++语言进行解答。这个资源包含大约150个不同的编程挑战,旨在帮助程序员提升算法设计和问题解决能力。 【描述】提到的"POJ题解及题目分类"是一...
【标题】"poj acm 题解 算法"所指的是一份针对ACM(国际大学生程序设计竞赛)中POJ(Problemset Online Judge)平台上的题目进行解答的资源集合。ACM竞赛是全球范围内的一项编程竞赛,旨在提升大学生的算法设计和...
《北大ACM题解》是一本专为解决POJ(Programming Online Judge)平台上的编程竞赛题目而编写的指导书籍。ACM(国际大学生程序设计竞赛,International Collegiate Programming Contest)是一项全球性的比赛,旨在...
《POJ部分题解》 POJ,全称为Programming Online Judge,是一个著名的在线编程竞赛平台,主要面向全球的计算机科学爱好者和程序员,提供了一个检验和提升编程能力的环境。在这个平台上,用户可以尝试解决各种算法...
这些文件名揭示了若干个在编程竞赛中常见的算法和数据结构问题,主要集中在POJ(Programming Online Judge)和PKU(Peking University)的在线评测系统上。下面将逐一解析这些题目并介绍相关的编程知识点: 1. **...
Poj1155 题解 , 文档 , 资料
**标签**:“POJ 2411 题解” #### 二、题目描述与分析 题目名为“蒙德里安的梦”,要求使用2x1的小矩形去填充一个大矩形,并计算出所有可能的不同填充方式数量。这里的“不同”是指即使两种填充方式是对称的也被...
北京大学ACM题解训练指南是面向参与ACM国际大学生程序设计竞赛(ICPC)的学子们提供的一份宝贵资源。ACM竞赛旨在培养学生的算法设计、编程和问题解决能力,而这份指南则提供了大量经过精心挑选和解答的题目,帮助...
经典O(n)求最长回文 acmer新手可看
【标题】"POJ100题_C++_源码" 涉及的是C++编程语言在解决算法竞赛中的应用,尤其是针对POJ(Programming Online Judge)平台上的编程题目。POJ是一个在线的编程练习系统,它提供了一系列的算法问题供用户练习,提升...
【标题】"POJ2262-Goldbach's Conjecture"是一个编程竞赛题目,源自北京大学的在线判题系统POJ(Problem Online Judge)。这个题目是基于著名的数学猜想——哥德巴赫猜想(Goldbach's Conjecture)设计的。 【描述...
1000 1002 1003 1004 1005 1006 1007 1008 1011 1012 1013 1019 1028 1045 1046 1068 1080 1088 1163 1207 1218 1256 1298 1299 1316 1326 1401 1455 1477 1488 1503 1504 1517 1519 1547 1552 1565 1579 1607 1656 ...
### ACM POJ 1002题解摘要 #### 题目背景与目标 本题目来自POJ(Pacific OpenJudge)平台上的一个经典问题,编号为1002。题目要求解决的是电话号码标准化的问题,即如何将各种形式的电话号码转换成统一的标准格式...
《POJ2388-Who's in the Middle》是一个典型的计算机编程竞赛题目,主要考察参赛者的算法设计和实现能力。这个题目源自北京大学的在线评测系统POJ(PKU Online Judge),是编程爱好者和学生提升编程技能的重要平台之...
【标题】"POJ1696-Space Ant" 是一个来自北京大学在线判题系统POJ(Problem Set of Peking University)的编程题目。这个题目挑战程序员解决一个名为"Space Ant"的问题,它涉及到计算机科学中的算法设计和实现,特别...
【标题】"poj-2528 Mayor's posters 测试数据及答案"涉及的是一个编程竞赛中的问题,这个问题来源于POJ(Programming Online Judge)平台,编号为2528。POJ是一个在线的编程练习系统,它提供了一系列的问题供程序员...