Previous
Articles
New Features of WCF
4.0
: Part I
New Features of WCF 4.0
: Part
II
New Features of WCF 4.0
: Part III
New Features of WCF 4.0
: Part IV
Introduction
Microsoft.NET
4.0 and Visual Studio.NET 2010 ships a lot of new features in their
underlying technologies. In this series of articles, I want to talk
about the new features in the area of Windows Communication Foundation
(WCF) in order to improve the development experience, enable more
communication scenario, support new WS-* standards and provide a good
integration with Windows Workflow Foundation (WF).
The new
features are essentially the following: simplified configuration,
standard endpoints, IIS hosting without a SVC file, support for
WS-Discovery, routing service, REST improvements, enhancements for the
integration with WF to support workflow services, simple byte stream
encoding and ETW tracing.
In this series of article, I will
illustrate each feature explaining the principles and showing some
examples.
Workflow Services
Workflow Service is a
new approach to build integration solutions where we have a combination
of WCF and WF programming model and related technologies into one
paradigm. WF gives you a declarative programming model raising the level
of abstraction when exposing and consuming the business logic. This is a
very good technology to support the principles of an integration
solution where we specify the orchestration of services (functionality
exposed by the system to be integrated) as well as to expose this
orchestration (the implementation of a business logic) as service.
WF
provides a good model for implementing long-running orchestration that
need to wait for external events in an asynchronous way. So, the
combination of WCF and WF programming model and related technologies
enable the developers to have a very robust workflow technology such as
BizTalk Server or Oracle SOA. Of course, BizTalk Server and Oracle SOA
(Workflow Services is a not substitutive product but a complement) are
server solutions to execute orchestration in an enterprise environment
to guarantee the quality of services such as performance and
availability. The bottom line is we're replacing lines of code in the
implementation of the service operation with a composition of
activities.
The main artifacts to integrate WCF and WF in
Microsoft.NET 4.0 are the workflow services, the new class
WorkflowServiceHost and the set of WCF messaging and workflow
activities. Workflow services are defined in XAML files with a .xamlx
extension. These xamlx files contain the description of the workflow,
the activities and endpoint configurations not defined in the
configuration file.
In order to illustrate the concepts of
Workflow Services, I will develop a solution to support the simple
scenario where we receive a message, do some processing and send a
response back to the client. This scenario could be very complex such as
consuming external services, waiting for some events, and so on. The
implementation strategy is to use Workflow Services paradigm and new
features of WCF 4.0.
The first step is to open Visual Studio.NET
2010 and create a new project. In the New Project window, select the
Workflow node and then WCF Workflow Service Application template (see
Figure 1).
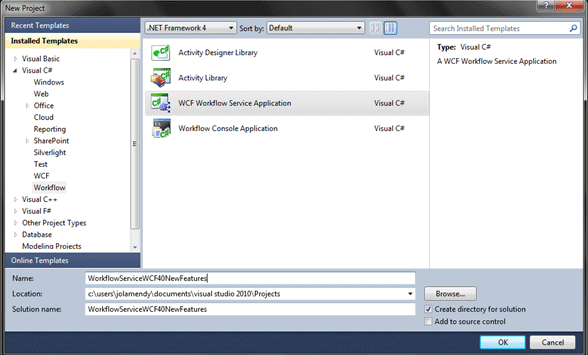
Figure 1
When the project is
created, we have mainly two files, the Service1.xaml with the definition
of the workflow and the Web.config with the application configuration
specifically with configuration of the WCF service with configurations
for endpoints, bindings and behaviors.
If you see the workflow
designer, by default, the workflow service contains a Receive activity
followed by a Send activity. The Receive activity enables that when one
incoming message is received, then a new instance of the workflow is
created. This behavior of configured by setting the CanCreateInstance
property of the Receive activity to true. The Receive activity contains
the GetData operation. You can change the name of the operation with
OperationName property(see Figure 2).
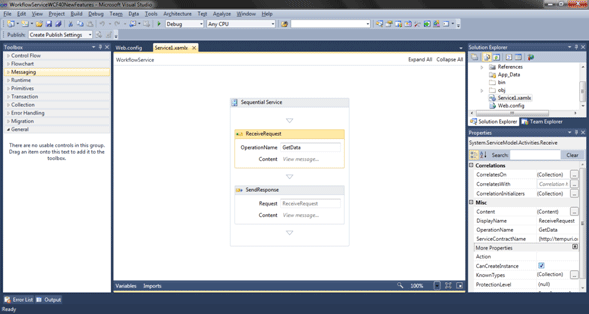
Figure 2
The Receive
activity also has the Content property for binding the incoming
messages to local variables within the Sequence activity (see Figure 2).
If you click on the Content property, the Content Definition window
appears, and you see that incoming messages are bound to the data
variable of type Int32 (see Figure 3).
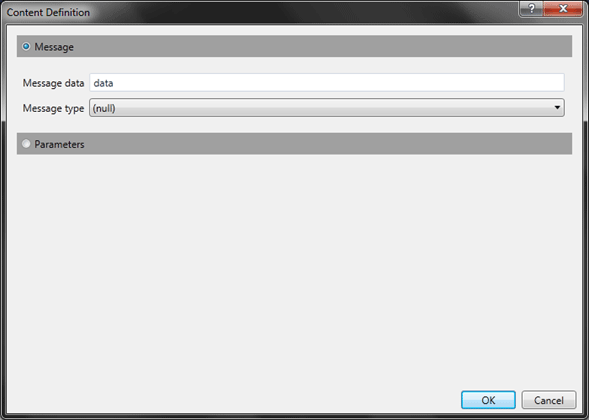
Figure
3
You can see the list of variables associated to the Sequence
activity by clicking on the Variables tab underneath the workflow
designer (see Figure 4).
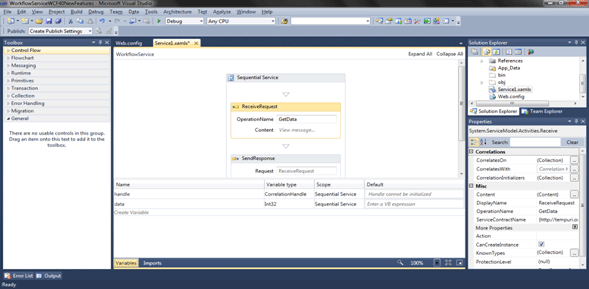
Figure
4
Let's create the EchoService as we've done in previous
articles of the series. First of all, let's rename the service from
Service1.xamlx to EchoService.xamlx in the Solution Explorer window as
well as the ConfigurationName and Name properties of the Service in the
Properties window (see Figure 5).
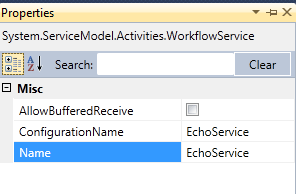
Figure
5
Next step is to change the name of the operation to GetMessage
and define a new variable named inMessage of type String within the
Sequence (see Figure 6).

Figure
6
Next step is to bind the Content property of the Receive
activity to the inMessage variable (see Figure 7).
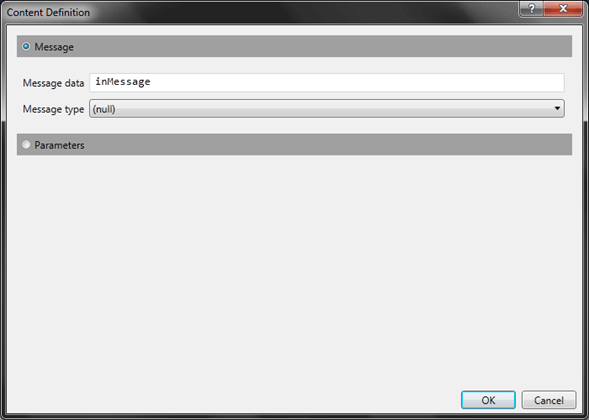
Figure
7
Next, let's bind the Content property of the Send activity to
the pattern "Echo Message is {0}" where the parameter is the inMessage
variable (see Figure 8).
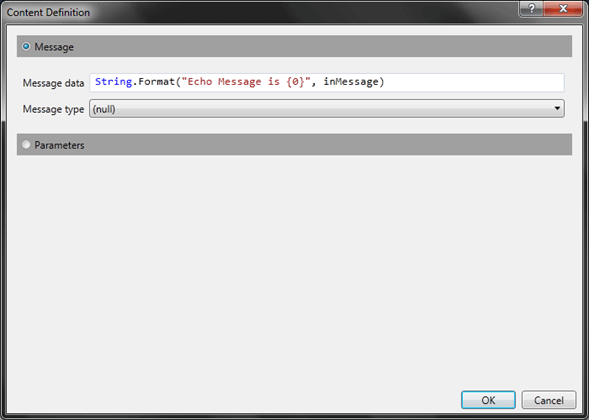
Figure
8
In order to test this service, press the F5 key and the
workflow service will be loaded in the ASP.NET Development Server and
the WCF Test Client application is also launched. The WCF Test Client
automatically connects to the service and uses the WSDL metadata to
create the client-side artifacts. You can see that the WSDL file is
generated by the Receive and Send activities in the workflow.
If
you double-click on the GetMessage operation a new tab appears to fill
the request data, then click on the Invoke button and see the results on
the Response pane (see Figure 9).
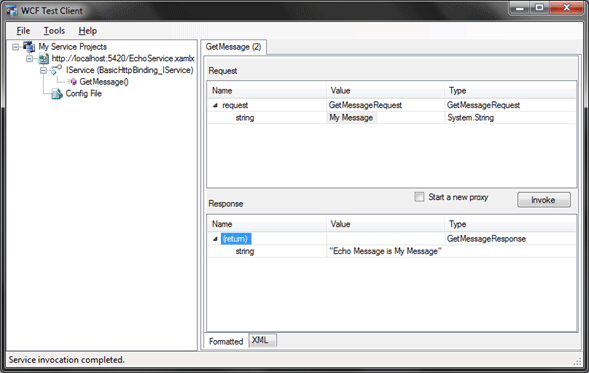
Figure
9
Let's send complex messages to the workflow service as it
would be in real scenarios. Let's define our complex user-defined data
contract to have two string properties to convey the message payload. We
need to add the EchoRequest class and a reference to the
System.Runtime.Serialization.dll assembly (see Listing 1).
using
System;
using
System.Collections.Generic;
using
System.Linq;
using
System.Web;
using
System.Runtime.Serialization;
namespace
WorkflowServiceWCF40NewFeatures
{
[DataContract
]
public
class
EchoRequest
{
[DataMember
]
public
string
MessagePart1 { get
; set
; }
[DataMember
]
public
string
MessagePart2 { get
; set
; }
}
}
Listing 1
Next step is to
define the echoRequest variable as type of EchoRequest data contract
(see Figure 10).

Figure
10
Now, let's bind the incoming messages to the echoRequest
variable using the Content property of the Receive activity (see Figure
11).
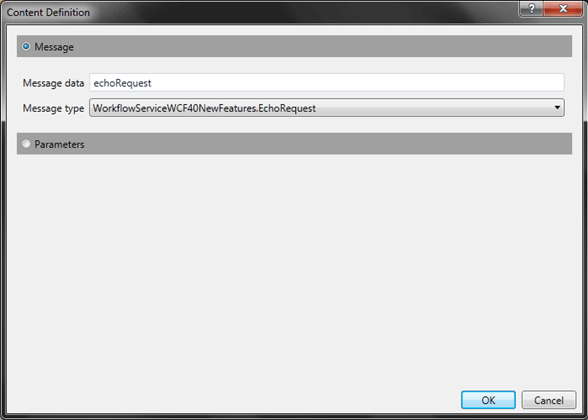
Figure
11
And let's change the output of the workflow service, by
changing the Content property of the SendReply activity (see Figure 12).
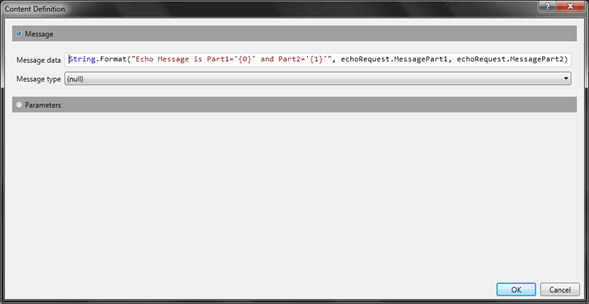
Figure
12
Let's run and test the workflow service (see Figure 13).
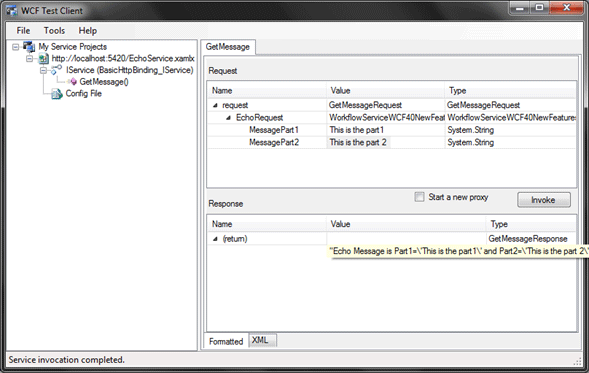
Figure
13
Now that we have our workflow service solution, we need to
host this service. You can host the service in the IIS (hosting a xamlx
service is identical to svc service) or self-hosted (in a standalone
process). It's remarkable to say that WCF 4.0 provides the needed
artifacts to support long-running orchestrations as well as for
correlating messages to the appropriate workflow service in the case of
having multiple service instances.
Hosting a workflow service in IIS
is identical to any WCF service. Indeed, we've tested the service in the
ASP.NET Development Server environment, so it would work the same way
in IIS environment. The bottom line is that your virtual directory
representing your Web application has to contain the xamlx file (with
the workflow definition), the web.config file and the bin directory
containing the assemblies with the custom types and activities used by
the workflow. In the web.config, you can configure any WCF endpoints and
behaviors.
Hosting a workflow service in a standalone
application is very simple too. You need to use the WorkflowServiceHost
class. Let's illustrate this scenario with an example by creating a
console application project and adding reference to the
System.Activities.dll, System.Xaml.dll, System.ServiceModel.dll and
System.ServiceModel.Activities.dll assemblies as well as to the project
done in the previous sections with the definitions of the workflow
services and related artifacts.
We also need to copy the xamlx
file from the workflow service project to this console project. The
reason is that workflow services are not compiled unlike the regular
workflows.
Now let's add the necessary code to host the workflow
service as shown in the Listing 2.
using
System;
using
System.Collections.Generic;
using
System.Linq;
using
System.Text;
using
System.ServiceModel;
using
System.ServiceModel.Description;
using
System.ServiceModel.Activities;
using
System.Xaml;
namespace
WorkflowServiceHostApp
{
class
Program
{
static
void
Main(string
[] args)
{
WorkflowServiceHost
serviceHost = new
WorkflowServiceHost
(XamlServices
.Load("EchoService.xamlx"
), new
Uri
("http://localhost:8080/Services/EchoService"
));
serviceHost.AddDefaultEndpoints();
serviceHost.Description.Behaviors.Add(new
ServiceMetadataBehavior
{
HttpGetEnabled=true
});
serviceHost.Open();
System.Console
.WriteLine("Press any key to finish ..."
);
System.Console
.ReadLine();
serviceHost.Close();
}
}
}
Listing 2
Conclusion
In
this series of article, I've explained the new features of WCF 4.0
through concepts and examples.
分享到:
相关推荐
根据提供的文件信息,我们可以归纳出一系列与WCF 4.0相关的专业知识点。这份教材主要围绕Windows Communication Foundation (WCF) 4.0展开,详细介绍了如何利用.NET 4进行服务开发、部署和服务交互等内容。下面我们...
### WCF 4.0 多层服务开发与 LINQ to Entities #### 一、WCF 4.0 概述 Windows Communication Foundation (WCF) 是 Microsoft 提供的一个用于构建服务导向架构(SOA)应用程序的框架。WCF 4.0 作为 .NET Framework...
Packtpub WCF 4.0 Multi tier Services Development with LINQ to Entities Jun.2010 关于WCF 4.0 和LINQ to Entity 的 新书 流行加时尚的编程利器
一个非常简单的WCF例子:Hello World 本解决方案有3个项目: 1、WCFClient:客户端,展示通过wcf后得到的结果,一个小的winform; 2、WCFContrlPanel:主控程序,作为打开/关闭wcf服务的控制面板,winform; 3、...
在技术层面,本书涵盖了WCF 4.0的核心部分,包括服务模型、宿主模型、绑定、消息交换模式、元数据发布、安全性、事务处理、消息路由、可靠性以及宿主和部署等。这些内容帮助读者构建和维护WCF服务,以及了解如何在...
6. **服务质量**:WCF提供服务质量管理,包括服务质量QoS(Quality of Service),如消息队列、负载均衡和故障恢复,确保服务的高效稳定运行。 7. **服务发现**:WCF有内置的服务发现机制,使得服务可以在网络中被...
【标题】"我的第一个WCF程序:HelloInDigo"是一个初学者的实践项目,它展示了如何使用Windows Communication Foundation(WCF)技术创建一个简单的服务。WCF是.NET框架的一部分,用于构建分布式、面向服务的应用程序...
**创建一个简单的WCF程序:WcfServices与WcfServices2** Windows Communication Foundation(WCF)是.NET Framework中用于构建分布式应用程序的一种强大的服务导向架构。它允许开发人员创建跨平台的、安全的、可靠...
WCF全面解析:上册.part3.rar
标题中的“Packtpub.WCF.4.0.Multi.tier.Services.Development.with.LINQ.to.Entities.Jun.2010”暗示了这是一份关于使用Windows Communication Foundation(WCF)4.0开发多层服务的教程,其中特别强调了使用LINQ to...
Windows Communication Foundation (WCF) 是微软推出的一种用于构建分布式应用程序的框架,它集成了多种通信技术,为开发者提供了一种统一的方式来创建、发布和消费服务。WCF是.NET Framework 3.0及更高版本的一部分...
WCF全面解析:上册.part2.rar
**WCF技术专题:WCF入门与进阶** Windows Communication Foundation(WCF)是微软推出的一种面向服务的架构,用于构建可互操作的分布式应用程序。它整合了.NET框架中的多种通信技术,如ASMX、Remoting、Web ...
《Wrox Professional WCF 4: Windows Communication Foundation with .NET 4》这本书是关于Windows Communication Foundation(WCF)技术的权威指南,专为.NET Framework 4.0设计。WCF是微软提供的一种全面的、统一...