- 浏览: 14233 次
- 性别:
- 来自: 郑州
-
最新评论
In this tutorial, we show you how to integrate AspectJ annotation with Spring AOP framework. In simple, Spring AOP + AspectJ allow you to intercept method easily.
Common AspectJ annotations :
- @Before – Run before the method execution
- @After – Run after the method returned a result
- @AfterReturning – Run after the method returned a result, intercept the returned result as well.
- @AfterThrowing – Run after the method throws an exception
- @Around – Run around the method execution, combine all three advices above.
For Spring AOP without AspectJ support, read this build-in Spring AOP examples.
1. Directory Structure
See directory structure of this example.
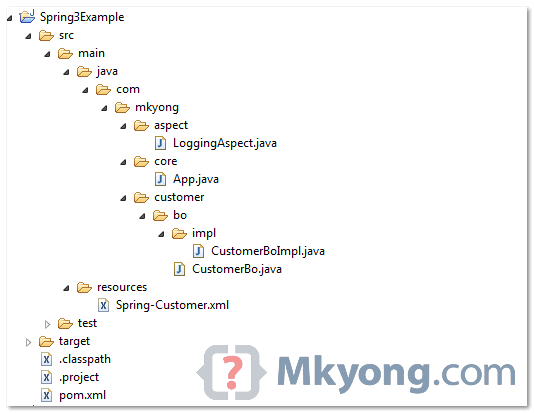
2. Project Dependencies
To enable AspectJ, you need aspectjrt.jar, aspectjweaver.jar and spring-aop.jar. See following Maven pom.xml
file.
This example is using Spring 3, but the AspectJ features are supported since Spring 2.0.
File : pom.xml
<project ...> <properties> <spring.version>3.0.5.RELEASE</spring.version> </properties> <dependencies> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-core</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>${spring.version}</version> </dependency> <!-- Spring AOP + AspectJ --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-aop</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.aspectj</groupId> <artifactId>aspectjrt</artifactId> <version>1.6.11</version> </dependency> <dependency> <groupId>org.aspectj</groupId> <artifactId>aspectjweaver</artifactId> <version>1.6.11</version> </dependency> </dependencies> </project>
3. Spring Beans
Normal bean, with few methods, later intercept it via AspectJ annotation.
package com.mkyong.customer.bo; public interface CustomerBo { void addCustomer(); String addCustomerReturnValue(); void addCustomerThrowException() throws Exception; void addCustomerAround(String name); }
package com.mkyong.customer.bo.impl; import com.mkyong.customer.bo.CustomerBo; public class CustomerBoImpl implements CustomerBo { public void addCustomer(){ System.out.println("addCustomer() is running "); } public String addCustomerReturnValue(){ System.out.println("addCustomerReturnValue() is running "); return "abc"; } public void addCustomerThrowException() throws Exception { System.out.println("addCustomerThrowException() is running "); throw new Exception("Generic Error"); } public void addCustomerAround(String name){ System.out.println("addCustomerAround() is running, args : " + name); } }
4. Enable AspectJ
In Spring configuration file, put “<aop:aspectj-autoproxy />
“, and define your Aspect (interceptor) and normal bean.
File : Spring-Customer.xml
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:aop="http://www.springframework.org/schema/aop" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-3.0.xsd "> <aop:aspectj-autoproxy /> <bean id="customerBo" class="com.mkyong.customer.bo.impl.CustomerBoImpl" /> <!-- Aspect --> <bean id="logAspect" class="com.mkyong.aspect.LoggingAspect" /> </beans>
4. AspectJ @Before
In below example, the logBefore()
method will be executed before the execution of customerBo interface, addCustomer()
method.
AspectJ “pointcuts” is used to declare which method is going to intercept, and you should refer to this Spring AOP pointcuts guide for full list of supported pointcuts expressions.
File : LoggingAspect.java
package com.mkyong.aspect; import org.aspectj.lang.JoinPoint; import org.aspectj.lang.annotation.Aspect; import org.aspectj.lang.annotation.Before; @Aspect public class LoggingAspect { @Before("execution(* com.mkyong.customer.bo.CustomerBo.addCustomer(..))") public void logBefore(JoinPoint joinPoint) { System.out.println("logBefore() is running!"); System.out.println("hijacked : " + joinPoint.getSignature().getName()); System.out.println("******"); } }
Run it
CustomerBo customer = (CustomerBo) appContext.getBean("customerBo"); customer.addCustomer();
Output
logBefore() is running! hijacked : addCustomer ****** addCustomer() is running
5. AspectJ @After
In below example, the logAfter()
method will be executed after the execution of customerBo interface, addCustomer()
method.
File : LoggingAspect.java
package com.mkyong.aspect; import org.aspectj.lang.JoinPoint; import org.aspectj.lang.annotation.Aspect; import org.aspectj.lang.annotation.After; @Aspect public class LoggingAspect { @After("execution(* com.mkyong.customer.bo.CustomerBo.addCustomer(..))") public void logAfter(JoinPoint joinPoint) { System.out.println("logAfter() is running!"); System.out.println("hijacked : " + joinPoint.getSignature().getName()); System.out.println("******"); } }
Run it
CustomerBo customer = (CustomerBo) appContext.getBean("customerBo"); customer.addCustomer();
Output
addCustomer() is running logAfter() is running! hijacked : addCustomer ******
6. AspectJ @AfterReturning
In below example, the logAfterReturning()
method will be executed after the execution of customerBo interface, addCustomerReturnValue()
method. In addition, you can intercept the returned value with the “returning” attribute.
To intercept returned value, the value of the “returning” attribute (result) need to be same with the method parameter (result).
File : LoggingAspect.java
package com.mkyong.aspect; import org.aspectj.lang.JoinPoint; import org.aspectj.lang.annotation.Aspect; import org.aspectj.lang.annotation.AfterReturning; @Aspect public class LoggingAspect { @AfterReturning( pointcut = "execution(* com.mkyong.customer.bo.CustomerBo.addCustomerReturnValue(..))", returning= "result") public void logAfterReturning(JoinPoint joinPoint, Object result) { System.out.println("logAfterReturning() is running!"); System.out.println("hijacked : " + joinPoint.getSignature().getName()); System.out.println("Method returned value is : " + result); System.out.println("******"); } }
Run it
CustomerBo customer = (CustomerBo) appContext.getBean("customerBo"); customer.addCustomerReturnValue();
Output
addCustomerReturnValue() is running logAfterReturning() is running! hijacked : addCustomerReturnValue Method returned value is : abc ******
7. AspectJ @AfterReturning
In below example, the logAfterThrowing()
method will be executed if the customerBo interface, addCustomerThrowException()
method is throwing an exception.
File : LoggingAspect.java
package com.mkyong.aspect; import org.aspectj.lang.JoinPoint; import org.aspectj.lang.annotation.Aspect; import org.aspectj.lang.annotation.AfterThrowing; @Aspect public class LoggingAspect { @AfterThrowing( pointcut = "execution(* com.mkyong.customer.bo.CustomerBo.addCustomerThrowException(..))", throwing= "error") public void logAfterThrowing(JoinPoint joinPoint, Throwable error) { System.out.println("logAfterThrowing() is running!"); System.out.println("hijacked : " + joinPoint.getSignature().getName()); System.out.println("Exception : " + error); System.out.println("******"); } }
Run it
CustomerBo customer = (CustomerBo) appContext.getBean("customerBo"); customer.addCustomerThrowException();
Output
addCustomerThrowException() is running logAfterThrowing() is running! hijacked : addCustomerThrowException Exception : java.lang.Exception: Generic Error ****** Exception in thread "main" java.lang.Exception: Generic Error //...
8. AspectJ @Around
In below example, the logAround()
method will be executed before the customerBo interface, addCustomerAround()
method, and you have to define the “joinPoint.proceed();
” to control when should the interceptor return the control to the original addCustomerAround()
method.
File : LoggingAspect.java
package com.mkyong.aspect; import org.aspectj.lang.ProceedingJoinPoint; import org.aspectj.lang.annotation.Aspect; import org.aspectj.lang.annotation.Around; @Aspect public class LoggingAspect { @Around("execution(* com.mkyong.customer.bo.CustomerBo.addCustomerAround(..))") public void logAround(ProceedingJoinPoint joinPoint) throws Throwable { System.out.println("logAround() is running!"); System.out.println("hijacked method : " + joinPoint.getSignature().getName()); System.out.println("hijacked arguments : " + Arrays.toString(joinPoint.getArgs())); System.out.println("Around before is running!"); joinPoint.proceed(); //continue on the intercepted method System.out.println("Around after is running!"); System.out.println("******"); } }
Run it
CustomerBo customer = (CustomerBo) appContext.getBean("customerBo"); customer.addCustomerAround("mkyong");
Output
logAround() is running! hijacked method : addCustomerAround hijacked arguments : [mkyong] Around before is running! addCustomerAround() is running, args : mkyong Around after is running! ******
Conclusion
It’s always recommended to apply the least power AsjectJ annotation. It’s rather long article about AspectJ in Spring. for further explanations and examples, please visit the reference links below.
文章来自:http://www.mkyong.com/spring3/spring-aop-aspectj-annotation-example/
- Spring3-AOP-AspectJ-Examples.zip (7.1 KB)
- 下载次数: 40
相关推荐
本实例将详细探讨如何通过注解(Annotation)来实现Spring AOP的方法拦截。 一、Spring AOP基础 Spring AOP是Spring框架的一部分,它提供了一种在运行时织入横切关注点(如日志、事务管理等)到目标对象的能力。AOP...
Spring AOP(面向切面编程)是Spring框架的重要组成部分,它提供了一种模块化和声明式的方式来处理系统中的交叉关注点问题,如日志、事务管理、安全性等。本示例将简要介绍如何在Spring应用中实现AOP,通过实际的...
本篇内容将对Spring AOP中基于AspectJ注解的使用进行总结,并通过实际案例进行解析。 首先,让我们理解AspectJ注解在Spring AOP中的核心概念: 1. **@Aspect**: 这个注解用于定义一个类为切面,这个类将包含切点和...
AspectJ是一个成熟的AOP框架,Spring在其AOP实现中整合了AspectJ,提供了更强大的面向切面编程能力。本篇文章将详细探讨在Spring 2.5中使用AspectJ进行AOP开发所需的知识点。 首先,我们需要理解AOP的核心概念: 1....
### Spring AOP面向方面编程原理:AOP概念详解 #### 一、引言 随着软件系统的日益复杂,传统的面向对象编程(OOP)逐渐暴露出难以应对某些横切关注点(cross-cutting concerns)的问题。为了解决这一挑战,面向方面编程...
标题提到的"Spring 使用AspectJ 实现 AOP之前置通知小例子",指的是利用AspectJ在Spring中实现AOP的一种特定类型的通知——前置通知(Before advice)。前置通知在目标方法执行之前运行,但不会阻止方法的执行。这种...
SpringBoot结合AspectJ实现SpringAOP拦截指定方法的知识点涵盖了多个方面,这包括Spring AOP的基本概念、SpringBoot的应用、切点(Pointcut)与通知(Advice)的定义、自定义注解以及AspectJ的使用。以下是这些知识...
Spring AOP(面向切面编程)是Spring框架的重要组成部分,它提供了一种模块化和声明式的方式来处理系统中的交叉关注点问题,如日志、事务管理等。在本主题中,我们将深入探讨Spring AOP的注解版,它是基于Java注解的...
Spring AOP(面向切面编程)是Spring框架的重要组成部分,它提供了一种模块化和声明式的方式来处理系统中的交叉关注点问题,如日志、事务管理等。在本示例中,我们将深入探讨如何在Maven项目中设置和使用Spring AOP...
Spring AOP,全称Aspect-Oriented Programming(面向切面编程),是Spring框架的一个重要组成部分。它提供了一种模块化和声明式的方式来处理系统中的交叉关注点,如日志、事务管理、性能监控等。在Spring AOP中,...
Spring AOP(面向切面编程)是Spring框架的重要组成部分,它提供了一种强大的方式来实现代码的横切关注点,如日志记录、事务管理、性能监控等。在这个"spring aop API示例"中,我们将深入探讨如何利用Spring AOP的四...
综上所述,要解决“java.lang.ClassNotFoundException: org.aspectj.lang.annotation.Around”错误,确保这三个jar包已经正确地添加到项目的类路径中,并且正确配置了Spring的AOP设置。通过这种方式,你可以充分利用...
在Spring框架中,AOP(面向切面编程)是一种强大的设计模式,它允许开发者将关注点分离,将横切关注点(如日志、事务管理)从核心业务逻辑中解耦出来。本篇我们将深入探讨如何使用注解的方式来实现Spring AOP开发。 ...
Spring AOP 1.0是Spring框架早期的一个版本,它引入了面向切面编程(Aspect Oriented Programming,AOP)的概念,使得开发者可以方便地实现横切关注点,如日志记录、事务管理、性能监控等,从而提高代码的可读性和可...
Spring AOP支持两种织入方式:编译时织入(通过AspectJ编译器)和运行时织入(通过代理)。 在这个实例中,我们将主要关注运行时织入,因为它更易于理解和实施。首先,我们需要定义一个切面类,这个类通常包含一个...
在Spring中,切面可以通过`@Aspect`注解的类来表示,如`org.springframework.aop.aspectj.annotation.AnnotationAspectAdapter`。 3. **通知(Advice)**:通知是切面中定义的行为,包括前置通知、后置通知、异常...
1. **启用AOP代理**:在Spring配置文件中,通过<aop:aspectj-autoproxy/>元素启用基于注解的AOP。 ```xml <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi=...
在Spring框架中,AOP(面向切面编程)是一种强大的设计模式,它允许开发者将关注点从业务逻辑中分离出来,比如日志记录、事务管理、权限检查等。Spring 2.0引入了基于注解的AOP配置,极大地简化了AOP的使用。这篇...
本篇文章将深入探讨如何利用Spring的@AspectJ注解来实现AOP,这是一个入门级别的例子,旨在帮助开发者理解并掌握这一关键特性。 首先,我们要明白什么是AOP。面向切面编程是一种编程范式,它允许程序员定义“切面”...