一个相对简单的例子,对学习对话框很有帮助:
1新建Win32项目,编写代码
2新建资源,添加ICON
如图:
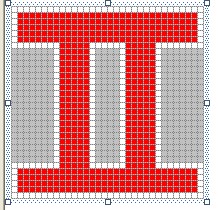
3资源,添加菜单:
如图:
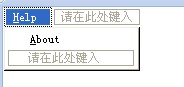
4资源,添加对话框,拖拽控件
如图:
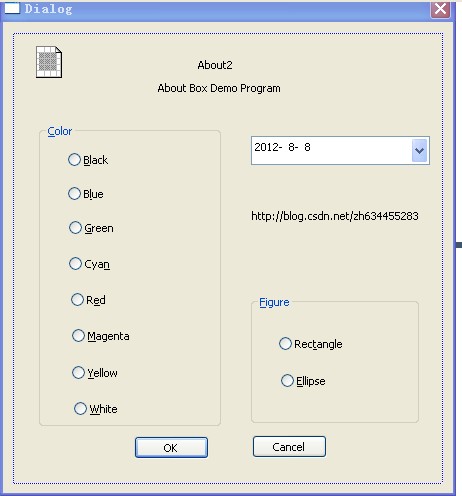
其中左上角的ICON就是刚刚建立的ICON:
即: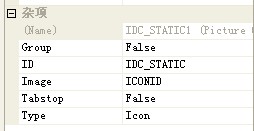
具体代码如下:
#include<windows.h>
#include"resource.h"
void PaintWindow(HWND hwnd,int iColor,int iFigure);//声明一个函数,这个函数主要是改变窗体背景颜色
BOOL CALLBACK DialogProc(HWND hwnd,UINT uMsg,WPARAM wParam,LPARAM lParam);//声明对话框过程函数
LRESULT CALLBACK WindowProc(
HWND hwnd, // handle to window
UINT uMsg, // message identifier
WPARAM wParam, // first message parameter
LPARAM lParam // second message parameter
);
int iCurrentColor=ID_BLACK;//声明两个全局变量,其中一个保存当前画刷的颜色
int iCurrentFigure=ID_RECTANGLE;//全局变量,保存当前的选中的图形
int WINAPI WinMain(
HINSTANCE hInstance, // handle to current instance
HINSTANCE hPrevInstance, // handle to previous instance
LPSTR lpCmdLine, // command line
int nCmdShow // show state
)
{
static TCHAR szAppName[]=TEXT("leidemingzi");
HWND hwnd;
MSG msg;
WNDCLASS wndclass;
HMENU hMenu;
wndclass.cbClsExtra=0;
wndclass.cbWndExtra=0;
wndclass.hbrBackground=(HBRUSH)GetStockObject(WHITE_BRUSH);
wndclass.hCursor=LoadCursor(NULL,IDC_ARROW);
wndclass.hIcon=LoadIcon(NULL,IDI_ERROR);
wndclass.hInstance=hInstance;
wndclass.lpfnWndProc=WindowProc;
wndclass.lpszClassName=szAppName;
wndclass.lpszMenuName=NULL;
wndclass.style=CS_HREDRAW|CS_VREDRAW;
if(!RegisterClass(&wndclass))//注册窗口
{
MessageBox(NULL,TEXT("the program require the window nt"),TEXT("tips"),MB_ICONERROR);
return 0;
}
hMenu=LoadMenu(hInstance,MAKEINTRESOURCE(MENUID));//加载菜单
hwnd=CreateWindow(
szAppName, // registered class name
TEXT("this is title"), // window name
WS_OVERLAPPEDWINDOW, // window style
CW_USEDEFAULT, // horizontal position of window
CW_USEDEFAULT, // vertical position of window
CW_USEDEFAULT, // window width
CW_USEDEFAULT, // window height
NULL, // handle to parent or owner window
hMenu, // menu handle or child identifier
hInstance, // handle to application instance
NULL // window-creation data
);
ShowWindow(hwnd,nCmdShow);
UpdateWindow(hwnd);
while(GetMessage(&msg,NULL,0,0))
{
TranslateMessage(&msg);
DispatchMessage(&msg);
}
return msg.wParam;
}
LRESULT CALLBACK WindowProc(
HWND hwnd, // handle to window
UINT uMsg, // message identifier
WPARAM wParam, // first message parameter
LPARAM lParam // second message parameter
)
{
static HINSTANCE hInst;
PAINTSTRUCT ps;
switch(uMsg)
{
case WM_CREATE:
hInst=((LPCREATESTRUCT)lParam)->hInstance;
return 0;
case WM_COMMAND:
switch(LOWORD(wParam))
{
case ID_HELP_ABOUT:
DialogBox(hInst,MAKEINTRESOURCE(DIALOGID),hwnd,DialogProc);//显示对话框
break;
}
return 0;
case WM_PAINT:
BeginPaint(hwnd,&ps);
PaintWindow(hwnd,iCurrentColor,iCurrentFigure);
MessageBeep(0);
EndPaint(hwnd,&ps);
return 0;
case WM_DESTROY:
PostQuitMessage(0);
return 0;
}
return DefWindowProc(hwnd,uMsg,wParam,lParam);
}
void PaintWindow(HWND hwnd,int iColor,int iFigure)
{
static COLORREF color[8]={RGB(0,0,0),RGB(0,0,255),RGB(0,255,0),RGB(0,255,255)//定义颜色数组,8种颜色,刚好对应单选按钮的颜色
,RGB(255,0,0),RGB(255,0,255),RGB(255,255,0),RGB(255,255,255)};
HDC hdc;
RECT rect;
hdc=GetDC(hwnd);
GetClientRect(hwnd,&rect);
HBRUSH hbrush=CreateSolidBrush(color[iColor-ID_BLACK]);//根据传进来的参数,创建画刷
SelectObject(hdc,hbrush);//把画刷选入环境设备中去
if(iFigure==ID_RECTANGLE)
{
Rectangle(hdc,rect.left,rect.top,rect.right,rect.bottom);//绘画矩形,自动填充颜色
}else
{
Ellipse(hdc,rect.left,rect.top,rect.right,rect.bottom);//绘画椭圆,自动填充颜色
}
ReleaseDC(hwnd,hdc);
}
void Flush(HWND hwnd)//这个方法是使客户区失效,从而触发WM_PAINT时间,不能直接在DialogProc使用InvaidateRect
//因为DialogProc中的hwnd指的是Dialog的句柄
{
InvalidateRect(hwnd,NULL,TRUE);
}
BOOL CALLBACK DialogProc(HWND hwnd,UINT uMsg,WPARAM wParam,LPARAM lParam)
{
//static HWND hCtrlBlock;
static int iColor,iFigure;
switch(uMsg)
{
case WM_INITDIALOG:
iColor=ID_WHITE;
iFigure=ID_RECTANGLE;
return TRUE;
case WM_COMMAND:
switch(LOWORD(wParam))//低位的wParam是ID
{
case IDOK:
iCurrentColor=iColor;
iCurrentFigure=iFigure;
//InvalidateRect(hwnd,NULL,TRUE);这是错误的,该hwnd是Dialog的句柄
Flush(GetParent(hwnd));
EndDialog(hwnd,0);
//MessageBeep(0);当时是用来测试用的,这个方法不错。
return TRUE;
case IDCANCEL:
EndDialog(hwnd,0);
return TRUE;
case ID_BLACK:
case ID_BLUE:
case ID_GREEN:
case ID_CYAN:
case ID_RED:
case ID_MAGENTA:
case ID_YELLOW:
case ID_WHITE:
iColor=LOWORD(wParam);
CheckRadioButton(hwnd,ID_BLACK,ID_WHITE,LOWORD(wParam));//切换按钮状态
//MessageBeep(0);
return TRUE;
case ID_RECTANGLE:
case ID_ELLIPSE:
iFigure=LOWORD(wParam);
CheckRadioButton(hwnd,ID_RECTANGLE,ID_ELLIPSE,LOWORD(wParam));//切换按钮状态
//MessageBeep(0);
return TRUE;
}
break;
}
return FALSE;
}
看看运行结果如何:
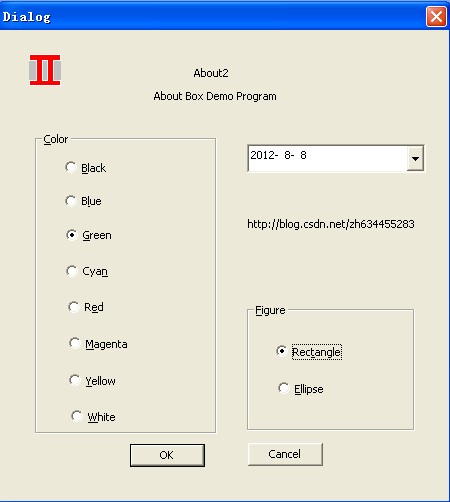
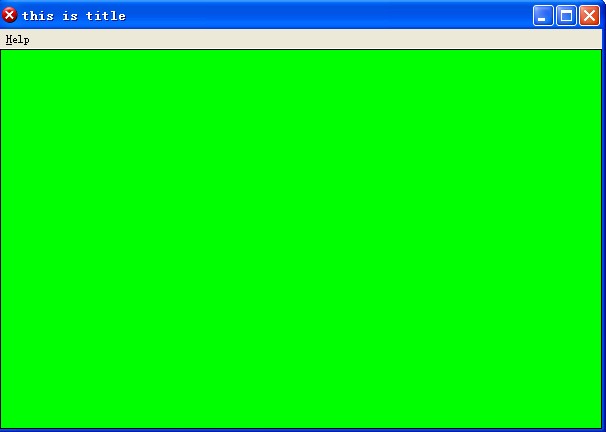
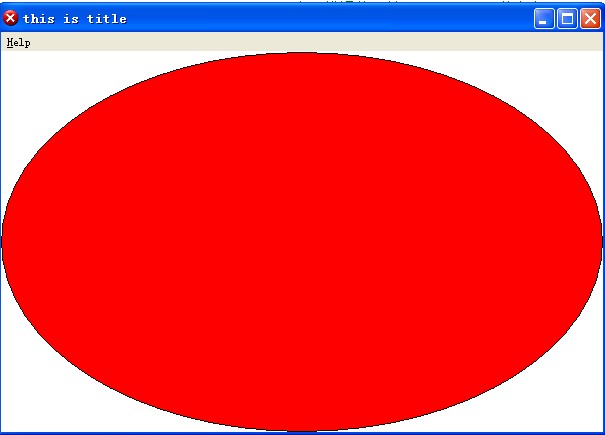
代码中有解释了,其中有一些很有用的函数,自己琢磨琢磨
分享到:
相关推荐
窗口悬浮置顶对话框是一种常见的用户界面设计技术,它允许特定的窗口或对话框始终位于其他应用程序窗口之上,保持在用户的视线范围内。这种设计通常用于提醒、通知或者需要用户即时关注的功能,例如音乐播放器的控制...
在IT领域的C++编程中,对窗口操作与鼠标控制是一项基本但重要的技能,尤其是在图形用户界面(GUI)应用开发中。本文将详细解析如何在MFC框架下实现窗口置顶功能以及如何限制鼠标在特定区域内的移动,这些技巧对于...
在Windows编程中,实现窗口置顶,即让一个窗口始终显示在其他窗口之上,是一种常见的需求,尤其是在开发桌面工具或应用程序时。这个“VC窗口置顶程序例子”提供了一个具体的解决方案,利用Microsoft Visual C++(VC...
在本文中,我们将深入探讨如何使用Microsoft Foundation Class (MFC) 库来创建一个具有特殊特性的窗口:无边框、可缩放且能够置顶显示。MFC 是微软为 Windows 开发应用程序提供的一种 C++ 类库,它封装了 Windows ...
在上述的`test0428`压缩包文件中,可能包含了一个简单的示例程序,演示了如何在对话框程序中创建和管理控制台。你可以解压后查看源代码,学习如何实际应用这些技术。通过这种方式,你可以让对话框程序具备控制台功能...
`GWL_EXSTYLE`用于获取或设置一个窗口的扩展样式,而`GWL_STYLE`则用于获取或设置窗口的基本样式。在MFC中,我们可以通过`GetWindowLong`函数获取这些样式,通过`SetWindowLong`函数进行设置。为了实现半透明效果,...
这是一个非常实用的功能,因为它允许用户直接在WinCC环境中选择需要的文件,方便了数据的导入导出以及相关操作。 文件中还附有操作截图,帮助用户直观理解每一步的具体操作和结果。文档最后还提供了西门子自动化...
用户只需要将工具提供的图标(通常是一个小的浮动窗口或系统托盘图标)从任务栏或者桌面拖动到目标窗口上,目标窗口就会被设置为置顶状态。这种交互方式简单易用,不需要用户进行复杂的设置或者记忆快捷键。 在实际...
通过以上步骤,我们可以实现一个基本的跟随置顶导航条。在实际项目中,可能还需要考虑更多细节,如动画效果、动态计算导航条高度、响应式设计等。此外,市面上也有许多现成的jQuery插件,如`sticky-kit`或` ...
本资源包含两个`.exe`文件,它们是独立的应用程序,用于实现这一功能。首先,我们需要理解窗口置顶的概念以及如何在Windows操作系统中实现这一功能。 窗口置顶是指将某个窗口设置为始终显示在其他窗口之上,即使...
【VC对话框隐藏运行--悬浮窗口】是一种在VC++编程中实现特定用户界面效果的技术,主要是创建一个没有边框、可以自由移动并且在任务栏中不显示的小窗口,通常用于提供快捷操作或辅助功能。本篇文章将详细讲解如何在...
嵌入式Android项目设计与开发 第四章 基本控件编程 ——警告对话框 警告对话框 AlertDialog可以在当前的界面弹出一个对话框,这个对话框是置顶于所有界面元素上,能够屏蔽掉其他控件的交互能力。 因此,AlertDialog...
Unity-置顶OpenFileDialog文件选择框 效果展示:https://blog.csdn.net/qq_26318597/article/details/134978622
在IT领域,创建一个基于对话框的日历应用是一项常见的任务,尤其对于桌面应用程序开发者来说。这个特定的应用程序设计包括几个关键的技术元素,如椭圆透明对话框、获取系统时间、对话框置顶以及显示详细的时间信息...
2. `SetWindowPos`函数:这是另一个重要的函数,它允许我们调整窗口的位置和大小,以及设置窗口的一些属性,比如置顶属性。通过传递特定的参数,我们可以将指定窗口设置为顶级窗口,使其始终位于其他窗口之上。在...
系统对话框在Windows编程中起着至关重要的作用,它们为用户提供了一种标准的界面来执行常见的任务,如打开或保存文件、设置颜色或字体等。以下是一些主要的系统对话框类型及其相关的WIN32API函数: 1. **Open/...
窗口置顶是一种系统功能,允许用户指定一个窗口始终保持在其他窗口之上显示。在Windows操作系统中,虽然原生并不提供此功能,但可以通过第三方软件实现。这两款软件就是这样的实用工具。 第一款置顶软件可能是...
2. **创建自定义窗体**:然后,创建一个新的`Form`实例,并将其`Opacity`属性设置为适当的值(比如0.9),使得背景看起来像是透明的。同时,将这个窗体的`TopMost`属性设置为`True`,确保它总是在其他窗口之上。 3....
ialogMate 提供4个帮助日常工作的视窗增强功能。 一、使用它你可以最小化窗口为悬浮图标(独特功能)以释放任务栏和托盘的空间。 二、集成到标题栏的小图标及窗口最小化及还原按钮,只需右击就能最小化窗口到托盘或...