Connection Modes
The following connection modes are supported:
- Embedded mode (local connections using JDBC)
- Remote mode (remote connections using JDBC or ODBC over TCP/IP)
- Mixed mode (local and remote connections at the same time)
Embedded Mode
In embedded mode, an application opens a database from within the same JVM using JDBC. This is the fastest and easiest connection mode. The disadvantage is that a database may only be open in one virtual machine (and class loader) at any time. As in all modes, both persistent and in-memory databases are supported. There is no limit on the number of database open concurrently, or on the number of open connections.

Remote Mode
When using the remote mode (sometimes called server mode or client/server mode), an application opens a database remotely using the JDBC or ODBC API. A server needs to be started within the same or another virtual machine (or on another computer). Many applications can connect to the same database at the same time. The remote mode is slower than the embedded mode, because all data is transferred over TCP/IP. As in all modes, both persistent and in-memory databases are supported. There is no limit on the number of database open concurrently, or on the number of open connections.
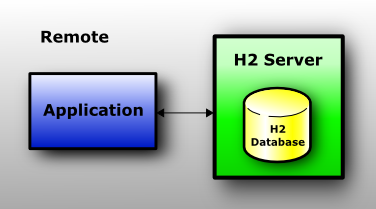
Mixed Mode
The mixed mode is a combination of the embedded and the remote mode. The main application connects to a database in embedded mode, but also starts a server so that other applications (running in different virtual machines) can concurrently access the same data. The embedded connections are as fast as if the database is used in just the embedded mode, while the remote connections are a bit slower.
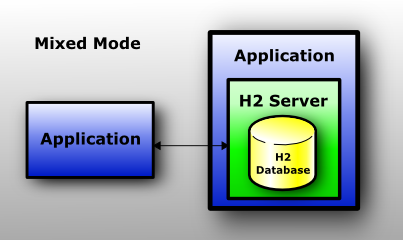
Mixed Mode Deploy
Method 1: Start server in J2EE application
Java Code (MixedMode.java):
package org.h2.samples;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
import java.sql.Statement;
import org.h2.tools.Server;
/**
* This sample program opens the same database once in embedded mode,
* and once in the server mode. The embedded mode is faster, but only
* the server mode supports remote connections.
*/
public class MixedMode {
/**
* This method is called when executing this sample application from the
* command line.
*
* @param args the command line parameters
*/
public static void main(String[] args) throws Exception {
// start the server, allows to access the database remotely
Server server = Server.createTcpServer(new String[] { "-tcpPort", "9101" });
server.start();
System.out.println("You can access the database remotely now, using the URL:");
System.out.println("jdbc:h2:tcp://localhost:9081/~/test (user: sa, password: sa)");
// now use the database in your application in embedded mode
Class.forName("org.h2.Driver");
Connection conn = DriverManager.getConnection("jdbc:h2:file:E:/Products/Opensource/h2/lee_test/test", "sa", "");
//Connection conn = DriverManager.getConnection("jdbc:h2:file:E:/Products/Opensource/h2/lee_test/test;AUTO_SERVER=TRUE", "sa", "");
// some simple 'business usage'
Statement stat = conn.createStatement();
stat.execute("DROP TABLE TIMER IF EXISTS");
stat.execute("CREATE TABLE TIMER(ID INT PRIMARY KEY, TIME VARCHAR)");
System.out.println("Execute this a few times: SELECT TIME FROM TIMER");
System.out.println("To stop this application (and the server), run: DROP TABLE TIMER");
try {
while (true) {
// runs forever, except if you drop the table remotely
stat.execute("MERGE INTO TIMER VALUES(1, NOW())");
Thread.sleep(1000);
}
} catch (SQLException e) {
System.out.println("Error: " + e.toString());
}
conn.close();
// stop the server
server.stop();
}
}
主程序Run后,是以Embeded模式连接,此时利用H2 Tools中的Broswer UI,进行连接(模拟另一Client),可以有以下几种连接方式:
1. jdbc:h2:tcp://localhost:9101/file:E:/Products/Opensource/h2/lee_test/test
2. jdbc:h2:tcp://localhost:9101/file:E:/Products/Opensource/h2/lee_test/test;AUTO_SERVER=TRUE
如果将程序中的黄底部分的语句换成绿底部分的语句,可以有以下几种连接方式:
1. jdbc:h2:tcp://localhost:9101/file:E:/Products/Opensource/h2/lee_test/test
2. jdbc:h2:tcp://localhost:9101/file:E:/Products/Opensource/h2/lee_test/test;AUTO_SERVER=TRUE
3. jdbc:h2:file:E:/Products/Opensource/h2/lee_test/test;AUTO_SERVER=TRUE (这种方式自动转换为Server模式,谁先连上谁是Embed模式)
Method 2: Start server via command line:
Command line:
@java -cp "h2.jar;%H2DRIVERS%;%CLASSPATH%" org.h2.tools.Server -tcp -tcpPort 9101
@if errorlevel 1 pause
Java Code (MixedMode.java):
package org.h2.samples;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
import java.sql.Statement;
import org.h2.tools.Server;
/**
* This sample program opens the same database once in embedded mode,
* and once in the server mode. The embedded mode is faster, but only
* the server mode supports remote connections.
*/
public class MixedMode {
/**
* This method is called when executing this sample application from the
* command line.
*
* @param args the command line parameters
*/
public static void main(String[] args) throws Exception {
// start the server, allows to access the database remotely
Server server = Server.createTcpServer(new String[] { "-tcpPort", "9101" });
server.start();
System.out.println("You can access the database remotely now, using the URL:");
System.out.println("jdbc:h2:tcp://localhost:9081/~/test (user: sa, password: sa)");
// now use the database in your application in embedded mode
Class.forName("org.h2.Driver");
//Connection conn = DriverManager.getConnection("jdbc:h2:file:E:/Products/Opensource/h2/lee_test/test", "sa", ""); //以Command Line方式启Server,不能使用这种方式了
Connection conn = DriverManager.getConnection("jdbc:h2:file:E:/Products/Opensource/h2/lee_test/test;AUTO_SERVER=TRUE", "sa", "");
// some simple 'business usage'
Statement stat = conn.createStatement();
stat.execute("DROP TABLE TIMER IF EXISTS");
stat.execute("CREATE TABLE TIMER(ID INT PRIMARY KEY, TIME VARCHAR)");
System.out.println("Execute this a few times: SELECT TIME FROM TIMER");
System.out.println("To stop this application (and the server), run: DROP TABLE TIMER");
try {
while (true) {
// runs forever, except if you drop the table remotely
stat.execute("MERGE INTO TIMER VALUES(1, NOW())");
Thread.sleep(1000);
}
} catch (SQLException e) {
System.out.println("Error: " + e.toString());
}
conn.close();
// stop the server
server.stop();
}
}
主程序Run后,是以Embeded模式连接,此时利用H2 Tools中的Broswer UI,进行连接(模拟另一Client),可以有以下几种连接方式:
1. jdbc:h2:file:E:/Products/Opensource/h2/lee_test/test;AUTO_SERVER=TRUE (这种方式自动转换为Server模式,谁先连上谁是Embed模式)
分享到:
相关推荐
【使用JAVA内存数据库h2database性能优化】 在开发应用程序时,我们经常遇到性能瓶颈,特别是当涉及到大量的IO操作时。数据库访问是这类问题的主要来源,特别是在处理高并发、实时计算和海量数据监控的情况下。例如...
H2就不做很多介绍了。资源包内容列表是我进行H2预研是收集的H2资料,应该是最全面的的了: ...10、H2内存数据库h2部署操作手册.docx 11、H2内存数据库安装与维护.doc 12、H2数据库基础知识.docx 13、H2数据库使用.doc
内存数据库H2 Database是Java开发的一个轻量级、高性能、开源的关系型数据库系统。它可以在内存中运行,也可以存储在磁盘上,并且支持多种模式,包括单用户模式、服务器模式以及分布式集群模式。H2 Database的设计...
内存数据库H2是一种轻量级、高性能的开源数据库系统,主要设计用于在内存中存储和处理数据,以提供快速的数据访问速度。它被广泛应用于测试、开发和嵌入式环境,尤其是在需要快速响应时间的应用中。H2数据库支持多种...
内存数据库,如H2,是一种将数据存储在内存中的数据库管理系统。相较于传统的磁盘存储数据库,内存数据库在处理速度上有着显著优势,因为它们避免了磁盘I/O操作的延迟。H2是一款开源、轻量级、高性能的数据库,常...
H2内存数据库h2部署操作手册.pdf
DBUnit与H2内存数据库结合是进行单元测试的一种高效方法,尤其在开发Java应用程序时,它可以帮助开发者确保数据层的功能正确性。这篇文章将详细介绍如何利用DBUnit和H2内存数据库来构建单元测试环境。 首先,DBUnit...
H2内存数据库是一种轻量级、高性能的关系型数据库,它主要设计用于嵌入式系统,也可作为服务器模式运行。H2数据库的特点在于其快速、小巧且完全免费,它支持多种数据库模式,包括单用户模式、多用户模式以及内存模式...
IT资料 常用软件 内存数据库 jar包
H2Database是一款轻量级、高性能的开源Java内存数据库系统,它被广泛应用于测试、开发以及嵌入式应用中。由于其支持多种数据库模式,包括单用户模式、服务器模式和混合模式,使得H2Database在各种场景下都能灵活运用...
H2内存数据库是一款轻量级、高性能的Java数据库系统,专为嵌入式和服务器模式设计,广泛应用于测试、开发以及小型应用。它的主要特点在于数据存储在内存中,提供了极快的读写速度,使得它成为快速原型开发或者临时...
本文将深入探讨如何利用内存数据库H2来高效地实现这一目标。H2是一个轻量级、高性能的关系型数据库,它支持多种模式,包括单线程、多线程、服务器模式等,且完全用Java编写,方便集成到Java项目中。 首先,我们需要...
Spring Boot 配置内存数据库 H2 教程详解 本文主要介绍了使用 Spring Boot 配置内存数据库 H2 的详细教程。H2 是一个轻量级的关系型数据库,可以用作内存数据库,非常适合用于开发和测试环境。 为什么选择 H2 ...
**H2内存数据库详解** H2数据库是一款轻量级、高性能的开源数据库系统,尤其适合于小型项目、测试环境以及快速原型开发。它的设计目标是简单、高效,并且完全支持SQL标准,使得开发者在处理数据存储时能拥有高度的...
H2 Database是一款开源、轻量级且高性能的内存数据库系统,设计用于在Java环境中运行,同时也支持多种其他语言。这款数据库引擎以其灵活性、易用性和跨平台性而受到开发者的欢迎,尤其适合于嵌入式应用和快速原型...
3. **多种模式**: H2支持多种数据库模式,包括单用户模式、多用户模式(TCP服务器)、内存模式(所有数据都存储在内存中)以及混合模式,这使得它能够适应不同的应用场景。 4. **兼容性**: H2数据库设计时充分考虑...
"内存数据库"则意味着H2可以在内存中运行,提供高速的数据访问。 【描述】提到,H2数据库是最新的纯Java实现,这意味着它可以在任何支持Java的平台上运行,无需额外安装其他数据库软件。它的“支持集群”特性表明H2...
H2是一个开源的嵌入式数据库引擎,采用java语言编写,不受平台的限制,同时H2提供了一 个十分方便的web控制台用于操作和管理数据库内容。H2还提供兼容模式,可以兼容一些主 流的数据库,具有比较完备的数据库特性...
h2 数据库导入 MySQL 数据库 h2 数据库导入 MySQL 数据库是指将 h2 数据库中的数据导入到 MySQL 数据库中,以便更好地存储和管理数据。下面将详细介绍 h2 数据库导入 MySQL 数据库的步骤。 首先,需要创建一个 ...
H2是另一款高性能、多模式的Java数据库,可运行于内存或磁盘。H2提供了丰富的特性,如支持多种存储引擎、嵌入式和服务器模式,以及良好的兼容性。对于开发和测试来说,H2是一个理想的选择,但在高负载生产环境中...