Task : Implement a WCF Service that contains a method that counts the number of words in a given text. The WCF Service will be released in 2 phases. For the phase 1 release, the WCF Service should satisfy the conditions in the Phase 1 Specifications. For the second release, the service should satisfy both the phase 1 and phase 2 specifications.
Phase 1 Specification:
•Definition of a word: In phase 1, a word is defined as a sequence of case-insensitive characters between ‘a’ and ‘z’ or between ‘A’ and Z. Any non-alphabetic character must be considered as a separator. The system however should be able to support different word formats (not just alphabetic), which may be defined in the next phase.
•Definition of word count : When counting words, the system should consider case-insensitive matching. For example, “THE” and “the” are considered to be the same word.
•For example : Given the text “THE quick brown fox jumped over|the-lazy{ broWn,moon”, the output of the wcf method should be something like
(“the”, 2), (“quick”,1), (“brown”,2), (“fox”,1), (“jumped”,1), (“over”,1), (“lazy”,1), (“moon”,1)
•The WCF Service will be used in an intranet settings.
•For the phase 1 release, the service will be used to process short text (only a few kilobytes).
Phase 2 Specification:
•Implement another method that returns the count of a specific word. If a word is missing from the input text, the return value should be zero. For example, given the text “THE quick brown fox jumped over|the-lazy{ brOwN moon”, searching for the word “brown” should return 2. Searching for the word “globalblue” on the other hand should return zero.
•Add support for Alphanumeric words.
•Add support for processing large texts ( a few megabytes)
Notes:
•When designing the solution, use your knowledge on good object oriented design practices as well as its implications on performance, code readability, testability and extensibility.
The solution as below:
ServiceLib => IHello.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.ServiceModel;
namespace ServiceLib
{
[ServiceContract(SessionMode = SessionMode.Required)]
public interface IHello
{
[OperationContract(IsInitiating = true, IsTerminating = false)]
Dictionary<string, int> GetDictionaryWords(string inputText, string pattern);
[OperationContract(IsInitiating = false, IsTerminating = false)]
int FindDictionaryWord(string inputText);
}
}
ServiceLib => Hello.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.ServiceModel;
using System.Text.RegularExpressions;
namespace ServiceLib
{
[ServiceBehavior(InstanceContextMode = InstanceContextMode.PerSession)]
public class Hello : IHello
{
Dictionary<string, int> wordsCount = new Dictionary<string, int>();
public Dictionary<string, int> GetDictionaryWords(string inputText, string pattern)
{
string[] words = null;
words = Regex.Split(inputText, pattern, RegexOptions.IgnoreCase);
for (int i = words.GetLowerBound(0); i <= words.GetUpperBound(0); i++)
{
string tempWords = words[i].ToString().ToLower();
if (wordsCount.ContainsKey(tempWords))
{
wordsCount[tempWords] = wordsCount[tempWords] + 1;
}
else
{
wordsCount.Add(tempWords, 1);
}
}
return wordsCount;
}
public int FindDictionaryWord(string inputText)
{
string tempWords = inputText.ToString().ToLower();
if (wordsCount.ContainsKey(tempWords))
{
return wordsCount[tempWords];
}
else
{
return 0;
}
}
}
}
ServicesHost =>Hello.svc
<%@ ServiceHost Language="C#" Debug="true" Service="ServiceLib.Hello" %>
ServicesHost =>Web.config
<?xml version="1.0"?>
<configuration>
<system.serviceModel>
<behaviors>
<serviceBehaviors>
<behavior name="SessionManagementBehavior">
<serviceMetadata httpGetEnabled="true"/>
<serviceDebug includeExceptionDetailInFaults="true"/>
</behavior>
</serviceBehaviors>
</behaviors>
<services>
<service name="ServiceLib.Hello" behaviorConfiguration="SessionManagementBehavior">
<endpoint address="" binding="wsHttpBinding" contract="ServiceLib.IHello" bindingConfiguration="MtomBindingConfiguration"/>
</service>
</services>
<bindings>
<wsHttpBinding>
<binding name="MtomBindingConfiguration" messageEncoding="Mtom" maxReceivedMessageSize="1073741824" receiveTimeout="00:10:00">
<!--maxArrayLength -->
<readerQuotas maxArrayLength="1073741824" />
</binding>
</wsHttpBinding>
</bindings>
</system.serviceModel>
<system.web>
<compilation debug="true"/>
</system.web>
</configuration>
Client => Program.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using Client.AlphanumericServices;
namespace Client
{
class Program
{
static void Main(string[] args)
{
AlphanumericServices.HelloClient serviceClient = new HelloClient();
string pattern = "[^\\w]+";
//string input = "THE quick brown fox jumped over|the-lazy{ broWn,moon";
//for (int i = 0; i < 10000; i++)
//{
// input = input + " " + input;
//}
Console.WriteLine("Please input a string:");
string input = Console.ReadLine();
foreach (var pair in serviceClient.GetDictionaryWords(input.Trim(), pattern))
{
Console.WriteLine("{0}, {1}",
pair.Key,
pair.Value);
}
string searchWord = Console.ReadLine();
Console.WriteLine(serviceClient.FindDictionaryWord(searchWord.Trim()));
Console.ReadKey();
}
}
}
Client => app.config
<?xml version="1.0" encoding="utf-8" ?>
<configuration>
<system.serviceModel>
<bindings>
<wsHttpBinding>
<binding name="MtomBindingConfiguration" messageEncoding="Mtom" sendTimeout="00:10:00">
<!--maxArrayLength-->
<readerQuotas maxArrayLength="1073741824" />
</binding>
</wsHttpBinding>
</bindings>
<client>
<endpoint address="http://localhost:5119/Hello.svc" binding="wsHttpBinding"
bindingConfiguration="MtomBindingConfiguration" contract="AlphanumericServices.IHello"
name="WSHttpBinding_IHello">
<identity>
<userPrincipalName value="VictorZeng-PC\Victor Zeng" />
</identity>
</endpoint>
</client>
</system.serviceModel>
</configuration>
The results as below:
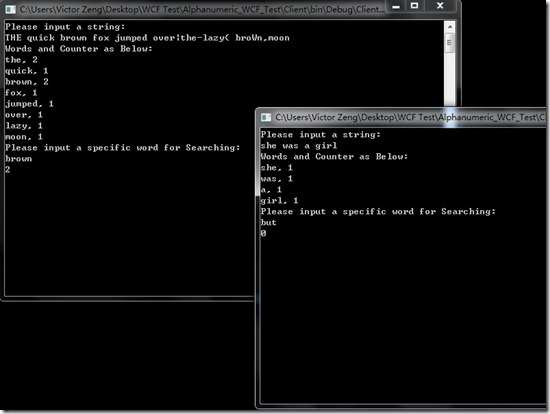
分享到:
相关推荐
【wcftest】是一个与C#编程相关的项目,很可能是一个用于测试Windows Communication Foundation(WCF)服务的工具或框架。Windows Communication Foundation是微软提供的一种框架,用于构建、配置和服务间通信的应用...
5. 官方和第三方的SDK、工具和库:例如,WCF Test Client可以帮助调试服务,NuGet包提供了额外的功能扩展。 通过这些资源,无论是理论学习还是实践经验,都可以帮助你更好地理解和掌握WCF,从而在SOA的世界里...
WCF服务是基于SOAP(Simple Object Access Protocol)协议的,可以与其他语言和平台进行交互。 2. JAVA调用WCF服务的必要条件 要调用WCF服务,需要满足以下条件: * 安装JDK和Eclipse开发环境 * 配置JAVA环境变量...
最常用的WebService协议是SOAP(Simple Object Access Protocol)和WSDL(Web Services Description Language),它们定义了如何封装、发送、接收数据以及如何描述服务。WebService通常使用HTTP协议进行传输,使得...
- **SOAP(Simple Object Access Protocol)**:一种基于XML的消息传输协议,常用于WCF服务的通信。 - **REST(Representational State Transfer)**:另一种常见的服务交互模型,以HTTP为基础,C#中的ASP.NET Web...
5. 集成测试:开发过程中,可以使用模拟Web服务(如WCF Test Client)来测试服务接口的正确性。 总结,.NET框架提供了强大的工具和技术来创建和使用Web服务,无论是ASMX的简单易用,还是WCF的灵活性和全面性,都能...
文件 "2016-11-04 Test"** 这个文件可能是一个源代码文件或者日志文件,与VS2015 Web服务项目相关。可能是测试用例、源代码示例或者服务运行时的日志记录。为了进一步了解其内容,需要查看文件的具体信息。 总结...
- 测试与调试:使用WCF Test Client工具或自行创建客户端应用程序来测试服务。 3. **调用Web Service** - SOAP请求:客户端通过构造符合SOAP规范的HTTP请求,发送到Web Service的URL来调用服务。 - WSDL文档:每...
Develop simple to complex user interfaces in Angular 4 Develop and handle forms in Angular 4 UI applications Test UIs built in Angular 4 Use material design components and animations in Angular 4 ...
源码可以帮助理解服务的内部工作原理,而工具如IDE插件(Eclipse的Web Service Explorer,Visual Studio的WCF Test Client)简化了测试过程。例如,`WebService.docx`可能包含一个简单的Web服务示例的源代码,展示了...