In this tutorial, we will show you how to use Maven to create a Java web application (with Spring MVC) project, and make it support Eclipse IDE.
Tools used :
- Maven 3.0.5
- Eclipse 4.2
- JDK 6
- Spring 3.2.0.RELEASED
- Tomcat 7
1. Web Application Project from Maven Template
In a terminal (*uix or Mac) or command prompt (Windows), navigate to the folder you want to store the project. Issue following command :
mvn archetype:generate -DgroupId={project-packaging} -DartifactId={project-name} -DarchetypeArtifactId=maven-archetype-webapp -DinteractiveMode=false
This tell Maven to create a Java web application project from “maven-archetype-webapp” template.
For example,
$ pwd /Users/mkyong/Documents/workspace $ mvn archetype:generate -DgroupId=com.mkyong -DartifactId=CounterWebApp -DarchetypeArtifactId=maven-archetype-webapp -DinteractiveMode=false [INFO] Scanning for projects... [INFO] [INFO] ------------------------------------------------------------------------ [INFO] Building Maven Stub Project (No POM) 1 [INFO] ------------------------------------------------------------------------ [INFO] [INFO] Generating project in Batch mode [INFO] ---------------------------------------------------------------------------- [INFO] Using following parameters for creating project from Old (1.x) Archetype: maven-archetype-webapp:1.0 [INFO] ---------------------------------------------------------------------------- [INFO] Parameter: groupId, Value: com.mkyong [INFO] Parameter: packageName, Value: com.mkyong [INFO] Parameter: package, Value: com.mkyong [INFO] Parameter: artifactId, Value: CounterWebApp [INFO] Parameter: basedir, Value: /Users/mkyong/Documents/workspace [INFO] Parameter: version, Value: 1.0-SNAPSHOT [INFO] project created from Old (1.x) Archetype in dir: /Users/mkyong/Documents/workspace/CounterWebApp [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 3.147s [INFO] Finished at: Thu Dec 20 20:35:19 MYT 2012 [INFO] Final Memory: 12M/128M [INFO] ------------------------------------------------------------------------
In above case, a new web application project named “CounterWebApp“, and the entire project directory structure is created automatically.
2. Maven Directory Layout
Maven created following web application directory layout. A standard deployment descriptor web.xml
and Maven pom.xml
are created.
CounterWebApp |-src |---main |-----resources |-----webapp |-------index.jsp |-------WEB-INF |---------web.xml |-pom.xml
Please check this official guide to understand more.
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.mkyong</groupId> <artifactId>CounterWebApp</artifactId> <packaging>war</packaging> <version>1.0-SNAPSHOT</version> <name>CounterWebApp Maven Webapp</name> <url>http://maven.apache.org</url> <dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>3.8.1</version> <scope>test</scope> </dependency> </dependencies> <build> <finalName>CounterWebApp</finalName> </build> </project>
<!DOCTYPE web-app PUBLIC "-//Sun Microsystems, Inc.//DTD Web Application 2.3//EN" "http://java.sun.com/dtd/web-app_2_3.dtd" > <web-app> <display-name>Archetype Created Web Application</display-name> </web-app>
<html> <body> <h2>Hello World!</h2> </body> </html>
3. Eclipse IDE Support
To convert the Maven web project to support Eclipse IDE, in terminal, navigate to “CounterWebAPp” folder, issue this command :
mvn eclipse:eclipse -Dwtpversion=2.0
You must add the -Dwtpversion=2.0
argument to make it as a Eclipse web project. Imports it into Eclipse IDE, a globe icon on top of project, means this is a web project in Eclipse.
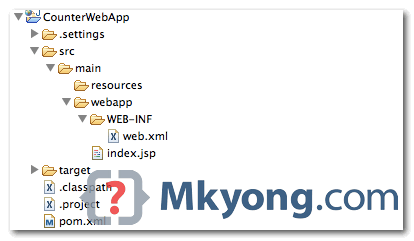
Many users are confused, again, if you just issue
mvn eclipse:eclipse
, it will only convert the project as Eclipse Java project, add extra -Dwtpversion=2.0
argument to make it as Eclipse web project.Done. This web project is ready to deploy. Attached to Eclipse’s Tomcat server plugin, and start it.

You can access the hello world jsp via – http://localhost:8080/CounterWebApp/
4. Update POM
To make above Maven web project to support Spring MVC framework, we need to touch up on the existing pom.xml
:
- Add compiler plugin to specify JDK6 to compile this project (default is using JDK1.4).
- Add Spring frameworks dependencies.
- Update jUnit to latest 4.11.
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.mkyong</groupId> <artifactId>CounterWebApp</artifactId> <packaging>war</packaging> <version>1.0-SNAPSHOT</version> <name>CounterWebApp Maven Webapp</name> <url>http://maven.apache.org</url> <properties> <spring.version>3.0.5.RELEASE</spring.version> <junit.version>4.11</junit.version> <jdk.version>1.6</jdk.version> </properties> <dependencies> <!-- Spring 3 dependencies --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-core</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-web</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-webmvc</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>${junit.version}</version> <scope>test</scope> </dependency> </dependencies> <build> <finalName>CounterWebApp</finalName> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.0</version> <configuration> <source>${jdk.version}</source> <target>${jdk.version}</target> </configuration> </plugin> </plugins> </build> </project>
5. Spring MVC REST
Create a Spring MVC controller class, with two simple methods to print a message.
package com.mkyong.controller; import org.springframework.stereotype.Controller; import org.springframework.ui.ModelMap; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; @Controller @RequestMapping("/") public class BaseController { @RequestMapping(value="/welcome", method = RequestMethod.GET) public String welcome(ModelMap model) { model.addAttribute("message", "Maven Web Project + Spring 3 MVC - welcome()"); //Spring uses InternalResourceViewResolver and return back index.jsp return "index"; } @RequestMapping(value="/welcome/{name}", method = RequestMethod.GET) public String welcomeName(@PathVariable String name, ModelMap model) { model.addAttribute("message", "Maven Web Project + Spring 3 MVC - " + name); return "index"; } }
Create a Spring configuration file, defines the Spring view resolver.
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:context="http://www.springframework.org/schema/context" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation=" http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.0.xsd"> <context:component-scan base-package="com.mkyong.controller" /> <bean class="org.springframework.web.servlet.view.InternalResourceViewResolver"> <property name="prefix"> <value>/WEB-INF/pages/</value> </property> <property name="suffix"> <value>.jsp</value> </property> </bean> </beans>
Update existing web.xml
to support Servlet 2.5 (the default Servlet 2.3 is too old), and also integrates Spring framework into this web application project via Spring’s listener ContextLoaderListener
.
<web-app xmlns="http://java.sun.com/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" version="2.5"> <display-name>Counter Web Application</display-name> <servlet> <servlet-name>mvc-dispatcher</servlet-name> <servlet-class> org.springframework.web.servlet.DispatcherServlet </servlet-class> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>mvc-dispatcher</servlet-name> <url-pattern>/</url-pattern> </servlet-mapping> <context-param> <param-name>contextConfigLocation</param-name> <param-value>/WEB-INF/mvc-dispatcher-servlet.xml</param-value> </context-param> <listener> <listener-class> org.springframework.web.context.ContextLoaderListener </listener-class> </listener> </web-app>
Move existing index.jsp
inside folder WEB-INF
, to protect user access it directly. In additional, edit the file to print out the${message}
variable that pass by the controller.
<html> <body> <h2>Hello World!</h2> <h4>Message : ${message}</h1> </body> </html>
Review the final directory structure

6. Eclipse + Tomcat
In order to start or debug this project via Eclipse server plugin (Tomcat or other container). You need to issue following command again, in order to make all dependencies attached to the project web deployment assembly.
mvn eclipse:eclipse -Dwtpversion=2.0
Before this command, project dependencies are empty.

After this command, now project dependdencies are here!

Many developers are trapped here, and failed to perform the starting or debugging in Eclipse server plugin, all failed by showing dependencies not found error message. Right click on your project properties, make sure all dependencies are inside the web deployment assembly, otherwise issue
mvn eclipse:eclipse -Dwtpversion=2.0
again!7. Maven Packaging
Review the pom.xml
again, the packaging
tag defining what is the packaging format or output.
<project ...> <modelVersion>4.0.0</modelVersion> <groupId>com.mkyong</groupId> <artifactId>CounterWebApp</artifactId> <packaging>war</packaging> <version>1.0-SNAPSHOT</version>
Package the project to deploy is easy, just issue mvn package
, it compiles and package the web project into a “war” file and store in project/target
folder.
For example :
$pwd /Users/mkyong/Documents/workspace/CounterWebApp Yongs-MacBook-Air:CounterWebApp mkyong$ mvn package [INFO] Scanning for projects... [INFO] [INFO] ------------------------------------------------------------------------ [INFO] Building CounterWebApp Maven Webapp 1.0-SNAPSHOT [INFO] ------------------------------------------------------------------------ [INFO] -- omitted for readability [INFO] [INFO] --- maven-war-plugin:2.1.1:war (default-war) @ CounterWebApp --- [INFO] Packaging webapp [INFO] Assembling webapp [CounterWebApp] in [/Users/mkyong/Documents/workspace/CounterWebApp/target/CounterWebApp] [INFO] Processing war project [INFO] Copying webapp resources [/Users/mkyong/Documents/workspace/CounterWebApp/src/main/webapp] [INFO] Webapp assembled in [87 msecs] [INFO] Building war: /Users/mkyong/Documents/workspace/CounterWebApp/target/CounterWebApp.war [INFO] WEB-INF/web.xml already added, skipping [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 3.936s [INFO] Finished at: Thu Dec 20 22:28:53 MYT 2012 [INFO] Final Memory: 14M/206M [INFO] ------------------------------------------------------------------------
Done, just copy the project/target/CounterWebApp.war
file and deploy to your container.
8. Demo
Start the web application.
http://localhost:8080/CounterWebApp/welcome

http://localhost:8080/CounterWebApp/welcome/mkyong

相关推荐
基于maven创建web项目 maven是一种基于项目对象模型(Project Object Model,POM)的项目管理工具,由Apache软件基金会开发和维护。maven提供了一种标准化的方式来构建、打包和部署项目,它可以帮助开发者简化项目...
本教程将指导您使用 IDEA 创建一个 Maven Web 项目,从安装 Maven 到配置 Maven 环境、创建 Maven 工程、pom.xml 文件配置、依赖管理等。 1. 安装 Maven 在 IDEA 中使用 Maven 之前,需要先安装 Maven。在 Maven ...
通过以上步骤,我们成功地使用IntelliJ IDEA 14.1.4和Maven创建了一个基本的Java Web项目。这个过程不仅让我们了解了IDEA和Maven的基本用法,也为今后的开发打下了坚实的基础。在实际开发过程中,还可以根据具体需求...
来自 在Eclipse中用Maven创建一个Web项目 的附带项目源码 jdk1.8 博客地址 https://blog.csdn.net/rebornsgundam/article/details/105862362
新版本IntelliJ IDEA 构建Maven,并用Maven创建一个Web项目的图文教程 Maven 项目基础知识 Maven 是一个基于项目对象模型(POM)的项目管理工具,由 Apache 软件基金会维护。Maven 的主要作用是管理项目的依赖关系...
【标题】:“idea使用maven创建的web项目” 在Java开发中,IntelliJ IDEA(简称Idea)作为一款强大的集成开发环境,结合Maven构建工具,能够帮助开发者高效地管理项目的依赖、构建和部署。Maven是一个项目管理和...
本文将深入探讨如何在Eclipse中利用Maven创建一个Web应用程序项目。 首先,我们需要理解“Eclipse使用Maven无法建web项目”这个问题可能涉及到的几个关键点。在Eclipse中创建Maven Web项目时,可能会遇到诸如Maven...
总结起来,通过Maven创建Web项目并配置Jetty运行涉及的关键步骤包括: 1. 编写`pom.xml`,添加必要的依赖和插件。 2. 编写或调整`web.xml`,定义Web应用的行为。 3. 可选地,根据需要调整`webdefault.xml`。 4. 使用...
1. **创建Maven Web项目**:使用Maven的archetype插件可以快速创建一个新的Web项目。例如,可以运行`mvn archetype:generate -DgroupId=...
通过以上步骤,我们成功地使用 Maven 和 Eclipse 创建了一个 Web 项目,并按照 Maven 的规范进行了结构调整。这种做法不仅有助于保持项目结构的清晰和一致,还能充分利用 Maven 的强大功能,提高开发效率。在未来...
如何在Eclipse IDE中使用maven创建一个动态Web项目. 使用的工具和技术 - Eclipse Jee Oxygen Maven 3.3.3 JavaSE 1.8 Servlet API 3.1.0 Apache Tomcat 7.0.47 (Embeded)
如何使用 Maven 创建一个 Java Web 项目(Spring MVC)。 用到的技术/工具: Maven 3.3.3 Eclipse 4.3 JDK 8 Spring 4.1.1.RELEASED Tomcat 7 Logback 1.0.13
在本文中,我们将深入探讨如何使用Maven创建一个多模块的Web项目,以及Maven的标准项目结构。Maven是一个强大的构建工具,它可以帮助开发者管理和构建Java项目。通过遵循Maven的标准项目对象模型(POM),我们可以...
标签"Maven Web"表示本文的主要内容是关于使用Maven创建Web项目的过程。 部分内容解释 一、建立Maven项目 建立Maven项目是创建基于Maven的Web项目的第一步。首先,选择建立Maven项目,选择File -> New -> Other,...
在创建Web项目时,还会包含`WEB-INF`目录,用于存放`web.xml`部署描述符以及Servlet相关的类等。 在这样一个项目中,开发者可能会涉及到的知识点包括: 1. Maven的生命周期与构建过程:理解`clean`、`compile`、`...
【标题】"mavenWeb空项目"所涉及的知识点主要集中在Java编程语言和Maven构建工具上,这是一个基于Maven的、已经过单元测试的Web应用程序的基础框架。下面将详细介绍这两个核心领域的相关知识。 **一、Java编程语言*...
在创建好 Maven Web 项目后,需要在 src/main/webapp/WEB-INF 下面创建一个 web.xml 文件,并在 pom.xml 文件中添加相应的依赖项。pom.xml 文件是 Maven 项目的核心配置文件,它定义了项目的依赖关系和构建过程。 ...
资源名称:maven创建web项目教程 中文WORD版内容简介: Maven项目对象模型(POM),可以通过一小段描述信息来管理项目的构建,报告和文档的软件项目管理工具。 Maven 除了以程序构建能力为特色之外,还提供高级项目...