转载:Spring Caching and Ehcache example
不过把ehcahce.xml中的updateCheck=true 改为 false
pom.xml中的log 的dependency 改为:
<!-- Optional, to log stuff --> <dependency> <groupId>org.slf4j</groupId> <artifactId>slf4j-log4j12</artifactId> <version>1.7.5</version> </dependency>
然后加上log4j.properties方便看log 输出:
log4j.rootLogger=debug,console log4j.appender.console=org.apache.log4j.ConsoleAppender log4j.appender.console.layout=org.apache.log4j.PatternLayout log4j.appender.console.layout.ConversionPattern=%d{yyyy-MM-dd HH:mm:ss} %-5p %c:%L - %m%n
In this tutorial, we will show you how to enable data caching in a Spring application, and integrate with the popular Ehcache framework.
Tools used
- Ehcache 2.9
- Spring 4.1.4.RELEASE
- Logback 1.0.13
- Maven 3 / Gradle 2
- JDK 1.7
- Eclipse 4.4
Spring supports caching since version 3.1
Spring cache has been significantly improved since version 4.1
1. Project Directory Structure
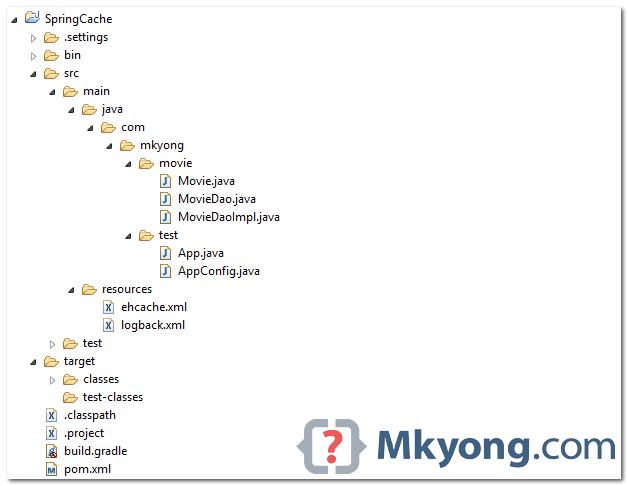
2. Project Dependencies
The Spring caching is in the spring-context.jar
, to support Ehcache caching, you need to include thespring-context-support.jar
as well.
For Maven project :
<dependency> <groupId>net.sf.ehcache</groupId> <artifactId>ehcache</artifactId> <version>2.9.0</version> </dependency> <!-- Optional, to log stuff --> <dependency> <groupId>ch.qos.logback</groupId> <artifactId>logback-classic</artifactId> <version>1.0.13</version> </dependency> <!-- Spring caching framework inside this --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>4.1.4.RELEASE</version> </dependency> <!-- Support for Ehcache and others --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context-support</artifactId> <version>4.1.4.RELEASE</version> </dependency> </project>
For Gradle project :
apply plugin: 'java' apply plugin: 'eclipse-wtp' version = '1.0' // Uses JDK 7 sourceCompatibility = 1.7 targetCompatibility = 1.7 // Get dependencies from Maven central repository repositories { mavenCentral() } //Project dependencies dependencies { compile 'org.springframework:spring-context:4.1.4.RELEASE' compile 'org.springframework:spring-context-support:4.1.4.RELEASE' compile 'net.sf.ehcache:ehcache:2.9.0' compile 'ch.qos.logback:logback-classic:1.0.13' }
3. Spring Non-Cache Example
A simple DAO to find a movie by director name.
package com.mkyong.movie; import java.io.Serializable; public class Movie implements Serializable { int id; String name; String directory; //getters and setters //constructor with fields //toString() }
package com.mkyong.movie; public interface MovieDao{ Movie findByDirector(String name); }
package com.mkyong.movie; import org.springframework.stereotype.Repository; @Repository("movieDao") public class MovieDaoImpl implements MovieDao{ //each call will delay 2 seconds, simulate the slow query call public Movie findByDirector(String name) { slowQuery(2000L); System.out.println("findByDirector is running..."); return new Movie(1,"Forrest Gump","Robert Zemeckis"); } private void slowQuery(long seconds){ try { Thread.sleep(seconds); } catch (InterruptedException e) { throw new IllegalStateException(e); } } }
package com.mkyong.test; import org.springframework.context.annotation.ComponentScan; import org.springframework.context.annotation.Configuration; @Configuration @ComponentScan({ "com.mkyong.*" }) public class AppConfig { }
package com.mkyong.test; import org.slf4j.Logger; import org.slf4j.LoggerFactory; import org.springframework.context.ApplicationContext; import org.springframework.context.annotation.AnnotationConfigApplicationContext; import com.mkyong.movie.MovieDao; public class App { private static final Logger log = LoggerFactory.getLogger(App.class); public static void main(String[] args) { ApplicationContext context = new AnnotationConfigApplicationContext(AppConfig.class); MovieDao obj = (MovieDao) context.getBean("movieDao"); log.debug("Result : {}", obj.findByDirector("dummy")); log.debug("Result : {}", obj.findByDirector("dummy")); log.debug("Result : {}", obj.findByDirector("dummy")); } }
Output
findByDirector is running... 2015-01-22 10:39:04 [main] DEBUG com.mkyong.test.App - Result : Movie [id=1, name=Forrest Gump, directory=Robert Zemeckis] findByDirector is running... 2015-01-22 10:39:06 [main] DEBUG com.mkyong.test.App - Result : Movie [id=1, name=Forrest Gump, directory=Robert Zemeckis] findByDirector is running... 2015-01-22 10:39:08 [main] DEBUG com.mkyong.test.App - Result : Movie [id=1, name=Forrest Gump, directory=Robert Zemeckis]
Each call to findByDirector
will take 2 seconds delay.
4. Spring Caching Example + EhCache
Now, we will enable data caching on method findByDirector
.
4.1 Create a ehcache.xml
file, to tell Ehcache how and where to cache the data.
<ehcache xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="ehcache.xsd" updateCheck="true" monitoring="autodetect" dynamicConfig="true"> <diskStore path="java.io.tmpdir" /> <cache name="movieFindCache" maxEntriesLocalHeap="10000" maxEntriesLocalDisk="1000" eternal="false" diskSpoolBufferSizeMB="20" timeToIdleSeconds="300" timeToLiveSeconds="600" memoryStoreEvictionPolicy="LFU" transactionalMode="off"> <persistence strategy="localTempSwap" /> </cache> </ehcache>
To learn how to configure Ehcache, read this official ehcache.xml example.
4.2 Add @Cacheable
on the method you want to cache.
package com.mkyong.movie; import org.springframework.cache.annotation.Cacheable; import org.springframework.stereotype.Repository; @Repository("movieDao") public class MovieDaoImpl implements MovieDao{ //This "movieFindCache" is delcares in ehcache.xml @Cacheable(value="movieFindCache", key="#name") public Movie findByDirector(String name) { slowQuery(2000L); System.out.println("findByDirector is running..."); return new Movie(1,"Forrest Gump","Robert Zemeckis"); } private void slowQuery(long seconds){ try { Thread.sleep(seconds); } catch (InterruptedException e) { throw new IllegalStateException(e); } } }
4.3 Enable Caching with @EnableCaching
and declared a EhCacheCacheManager
.
package com.mkyong.test; import org.springframework.cache.CacheManager; import org.springframework.cache.annotation.EnableCaching; import org.springframework.cache.ehcache.EhCacheCacheManager; import org.springframework.cache.ehcache.EhCacheManagerFactoryBean; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.ComponentScan; import org.springframework.context.annotation.Configuration; import org.springframework.core.io.ClassPathResource; @Configuration @EnableCaching @ComponentScan({ "com.mkyong.*" }) public class AppConfig { @Bean public CacheManager cacheManager() { return new EhCacheCacheManager(ehCacheCacheManager().getObject()); } @Bean public EhCacheManagerFactoryBean ehCacheCacheManager() { EhCacheManagerFactoryBean cmfb = new EhCacheManagerFactoryBean(); cmfb.setConfigLocation(new ClassPathResource("ehcache.xml")); cmfb.setShared(true); return cmfb; } }
4.4 In non-web application, you need to shut down the Spring context manually, so that Ehcache got chance to shut down as well, otherwise Ehcache manager will hang there.
package com.mkyong.test; import org.slf4j.Logger; import org.slf4j.LoggerFactory; import org.springframework.context.ApplicationContext; import org.springframework.context.ConfigurableApplicationContext; import org.springframework.context.annotation.AnnotationConfigApplicationContext; import com.mkyong.movie.MovieDao; public class App { private static final Logger log = LoggerFactory.getLogger(App.class); public static void main(String[] args) { ApplicationContext context = new AnnotationConfigApplicationContext(AppConfig.class); MovieDao obj = (MovieDao) context.getBean("movieDao"); log.debug("Result : {}", obj.findByDirector("dummy")); log.debug("Result : {}", obj.findByDirector("dummy")); log.debug("Result : {}", obj.findByDirector("dummy")); //shut down the Spring context. ((ConfigurableApplicationContext)context).close(); } }
Output
INFO: Initializing EhCache CacheManager findByDirector is running... 2015-01-22 10:53:28 [main] DEBUG com.mkyong.test.App - Result : Movie [id=1, name=Forrest Gump, directory=Robert Zemeckis] 2015-01-22 10:53:28 [main] DEBUG com.mkyong.test.App - Result : Movie [id=1, name=Forrest Gump, directory=Robert Zemeckis] 2015-01-22 10:53:28 [main] DEBUG com.mkyong.test.App - Result : Movie [id=1, name=Forrest Gump, directory=Robert Zemeckis] INFO: Shutting down EhCache CacheManager
Review the executed time, there is no delay. In addition, only one “findByDirector is running…” is printed, because this method only executed once, subsequent call will get the object from cache.
Done.
This article is to help you get started with Spring data caching, to learn more about other caching annotations like
@CacheEvict
, @CachePut
, @CacheConfig
and etc, please refer to this officialSpring Cache Abstraction documentation, quite detail over there.
相关推荐
在Spring中,我们可以通过`@Cacheable`、`@CacheEvict`和`@Caching`等注解来实现缓存功能。例如,在一个服务类中,我们可以这样使用: ```java import org.springframework.cache.annotation.Cacheable; import org...
在Spring 3.2版本中,Ehcache是一个常用的二级缓存框架,它与Spring的集成使得我们在开发过程中能够方便地实现数据缓存,提高应用性能。本文将详细讲解如何在Spring项目中通过注解来配置和使用Ehcache。 首先,我们...
在IT行业中,Spring框架是Java企业级应用开发的首选,而Ehcache则是一个流行的、高性能的缓存系统。本文将深入探讨如何将Ehcache与Spring进行整合,以提高应用程序的性能和效率,主要基于提供的"spring+ehcache"入门...
在本教程中,我们将深入探讨如何在Spring Boot项目中集成并使用Caching-EhCache来提升应用程序的性能。Spring Boot简化了配置过程,使得开发者能够快速地启用缓存功能,而EhCache作为一款广泛使用的Java缓存解决方案...
HTTP Support for Caching and Replication Section 5.1. Conditional Requests Section 5.2. Age and Expiration of Cached Objects Section 5.3. Request Redirection Section 5.4. Range Requests ...
2. **缓存注解**:Spring 提供了 `@Cacheable`、`@CacheEvict`、`@CachePut` 和 `@Caching` 等注解,用于标记方法,以便在执行前后自动进行缓存操作。`@Cacheable` 用于缓存方法的结果,`@CacheEvict` 用于清除缓存...
Spring Boot缓存示例我们将创建一个Web应用程序,以显示生命,宇宙和一切的答案。 由于这是一个相当复杂的问题,因此可能需要花费一些... $ spring init caching -d=web,freemarker,actuator,cacheUsing service at ...
Demo of ehCache distributed caching with terracotta in glassFish v3 可以参考:http://blog.csdn.net/guobin0719/archive/2011/04/25/6361940.aspx
Spring Recipes 3rd Edition Sources === These are the sources belonging to Spring Recipes 3rd Edition. Each chapter has its own sources and each chapter can contain ...19. Spring Caching 20. Grails
本篇文章将深入探讨如何使用注解配置Spring与EHCache或OSCache这两个流行的Java缓存解决方案。以下是对该主题的详细阐述: 1. **Spring缓存抽象** Spring 3.1引入了一种统一的缓存抽象,它允许开发者在不关心具体...
Database interaction using Spring and Hibernate/JPA- Spring Data JPA- Spring Data MongoDB- Messaging, emailing and caching support- Spring Web MVC- Developing RESTful web services using Spring Web ...
此外,Ehcache与Spring框架的集成也是常见的应用场景,通过Spring的缓存抽象,可以方便地将Ehcache集成到Spring应用中,实现声明式缓存管理。 总之,Ehcache是一个强大的缓存解决方案,通过`ehcache-core-2.5.2.jar...
- **分布式缓存(Distributed Caching)**:在多节点环境下,Ehcache支持分布式缓存,确保数据的一致性和高可用性。 - **缓存加载器(Cache Loader)**:当缓存中找不到数据时,可以通过缓存加载器从其他源(如...
Spring通过`@Cacheable`、`@CacheEvict`、`@Caching`等注解简化了缓存操作。例如: ```java @Service public class MyService { @Cacheable(value = "myCache", key = "#id") public Object getData(String id) { ...
#### 十一、Spring 缓存集成(Spring Caching with Ehcache) 1. **Spring Cache Abstraction**:介绍如何使用 Spring 的缓存抽象层来简化 Ehcache 的集成过程。 2. **注解驱动**:使用注解来标记需要缓存的方法。 ...
使用 Ehcache 三步搞定 Spring Boot 缓存的方法示例 Spring Boot 应用程序中,数据缓存是非常重要的一步骤,能够提高应用程序的性能和响应速度。本文主要介绍基于 Ehcache 3.0 来快速实现 Spring Boot 应用程序的...
Ehcache 3.x 引入了 Java Caching System (JSR 107) 规范,提供了更现代的 API 和更好的性能。 优化 Ehcache 包括调整缓存大小、设置合适的过期策略、监控缓存命中率、合理使用缓存分区等。 通过深入了解和实践 ...
在本文中,我们将深入探讨如何在Spring框架中集成并使用Ehcache作为缓存解决方案。SpringEhcacheOne项目展示了如何通过JavaConfig配置Spring来整合Ehcache,从而提高应用程序的性能和效率。 Ehcache是一款流行的...
在Spring Boot 2.0中,Ehcache是一个流行的、高性能的本地缓存解决方案,用于提升应用程序性能。本文将深入探讨如何在Spring Boot项目中集成并使用Ehcache进行数据缓存。 首先,Ehcache是由Talend公司维护的一个...
Spring3注解与Ehcache整合是现代Java应用中实现高效缓存管理的重要技术组合。在Spring框架中,注解提供了简洁的编程模型,而Ehcache则是一个广泛使用的开源缓存解决方案,它能有效提高应用程序性能,减少数据库访问...